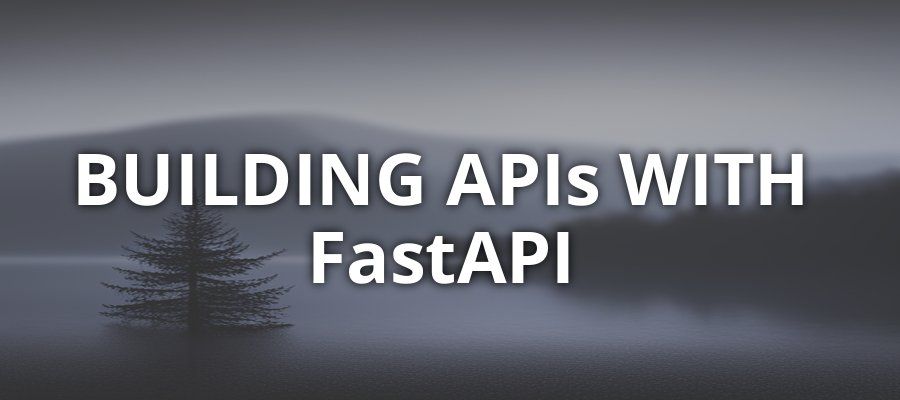
Building APIs with FastAPI
• December 29, 2023
FastAPI is an innovative, high-performance web framework for building REST APIs with Python 3.6+ based on standard Python type hints.
Introduction to FastAPI
FastAPI is an innovative, high-performance web framework for building APIs with Python 3.6+ based on standard Python type hints. The framework is designed to enable rapid development of robust APIs, while ensuring that the code remains clean and maintainable.
1.1 Understanding FastAPI and Its Ecosystem
FastAPI is built on top of Starlette for the web parts and Pydantic for the data parts. The combination of these tools provides a highly efficient and intuitive framework for web development. Starlette offers a lightweight ASGI framework/toolkit, which is ideal for building high-performance asyncio services. Pydantic, on the other hand, is a data validation and settings management library using Python type annotations.
The ecosystem around FastAPI includes various tools and integrations, making it a versatile choice for web API development. For instance, Uvicorn, an ASGI server, is commonly used to serve FastAPI applications, providing lightning-fast performance. The ecosystem also includes tools for interactive API documentation, such as Swagger UI and ReDoc, which are automatically generated by FastAPI.
1.2 Key Features and Advantages
FastAPI offers a plethora of features that cater to modern web development needs:
- Performance: Comparable to NodeJS and Go, FastAPI is one of the fastest web frameworks for Python, thanks to its underlying ASGI foundation.
- Type checks: Python type hints are not only used for type checking but also for API parameter definition, which simplifies the code and reduces bugs.
- Automatic documentation: With FastAPI, the interactive API documentation and exploration web user interfaces are generated automatically.
- Dependency injection: FastAPI includes a powerful dependency injection system, which is extremely easy to use.
- Security and authentication: The framework includes security and authentication as built-in features, supporting OAuth2 with Password (and hashing), including JWT tokens.
1.3 Installation and Setup
To install FastAPI, you need Python 3.6 or higher. The installation process is straightforward and can be done using pip, Python's package installer. Here's a basic guide to setting up a FastAPI environment:
pip install fastapi
pip install uvicorn[standard]
After installation, you can create a FastAPI instance and define endpoints. Here is a simple example of a FastAPI application:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def read_root():
return {"Hello": "World"}
To run the application, use Uvicorn as the ASGI server:
uvicorn main:app --reload
The --reload
flag enables auto-reload so the server will restart after code changes. This is useful during development but should be omitted in a production environment.
This section has provided an overview of FastAPI, its ecosystem, key features, and the initial steps to install and set up a FastAPI application. The subsequent sections will delve into building your first API with FastAPI, exploring advanced features, and discussing best practices for performance optimization, deployment, and monitoring.
Building Your First API with FastAPI
FastAPI is a modern, high-performance web framework for building APIs with Python 3.6+ based on standard Python type hints. The key features of FastAPI include its speed, ease of use, and automatic generation of interactive API documentation. This section will guide you through the creation of your first API using FastAPI, demonstrating the simplicity and power of the framework.
2.1 Creating a Basic API Endpoint
To create a basic API endpoint with FastAPI, you begin by importing the FastAPI
class and instantiating it as an app
object. This object is the main point of interaction to create all your API routes.
from fastapi import FastAPI
app = FastAPI()
Next, you define a route using one of the HTTP method decorators provided by FastAPI, such as @app.get("/")
. This decorator tells FastAPI that the following function is in charge of handling requests that go to the path /
using a GET
operation.
@app.get("/")
async def read_root():
return {"Hello": "World"}
The read_root
function is called whenever a GET
request is made to the root URL. It returns a JSON response containing a greeting. This is the simplest form of an API endpoint you can create with FastAPI.
2.2 Running the API with Uvicorn
Uvicorn is an ASGI server implementation that serves your FastAPI application. To run your application, you need to pass the app
instance you created to Uvicorn. This can be done using the command line:
uvicorn main:app --reload
The --reload
flag enables hot reloading, allowing the server to automatically restart upon code changes, which is very useful during development.
Once Uvicorn is running, it will display the URL where your API can be accessed, typically http://127.0.0.1:8000
. Visiting this URL in a web browser or using a tool like curl
will give you the JSON response from your read_root
function.
2.3 Exploring Interactive API Documentation
One of the standout features of FastAPI is the automatic generation of interactive API documentation using Swagger UI and ReDoc. Once your API is running, you can access these documentation interfaces by navigating to /docs
or /redoc
on your API's URL.
For example, if your API is running on http://127.0.0.1:8000
, you can find the Swagger UI documentation at http://127.0.0.1:8000/docs
and the ReDoc documentation at http://127.0.0.1:8000/redoc
.
These interactive tools provide a web-based interface to explore your API's routes, parameters, and responses. They also allow you to test API endpoints directly from the browser, making it easier to understand and use your API's capabilities.
By following these steps, you can build and run a simple API with FastAPI, leveraging its powerful features to create robust and well-documented web services.
Advanced FastAPI Features
In this section, we delve into the more sophisticated capabilities of FastAPI, a modern, fast web framework for building APIs with Python 3.7+ that is based on standard Python type hints. The focus here is on leveraging FastAPI's advanced features to enhance API functionality, enforce data integrity, and secure API endpoints.
3.1 Working with Path and Query Parameters
FastAPI provides a robust mechanism for defining path and query parameters that are essential for creating dynamic endpoints. Path parameters are used to capture values from the URL path, while query parameters are used to filter or customize responses based on the URL's query string.
from fastapi import FastAPI, Path, Query
app = FastAPI()
@app.get("/items/{item_id}")
async def read_item(item_id: int = Path(..., description="The ID of the item to retrieve"), q: str = Query(None, alias="item-query")):
return {"item_id": item_id, "q": q}
In the above snippet, item_id
is a path parameter that FastAPI validates to be of type int
, and q
is a query parameter with an alias item-query
. FastAPI automatically generates interactive API documentation that reflects these parameters.
3.2 Request Body and Data Validation with Pydantic
FastAPI integrates with Pydantic for data validation, serialization, and documentation of request bodies. Pydantic uses Python type annotations to validate the shape and content of incoming data, ensuring that the data conforms to specified schemas.
from fastapi import FastAPI
from pydantic import BaseModel
class Item(BaseModel):
name: str
description: str = None
price: float
tax: float = None
app = FastAPI()
@app.post("/items/")
async def create_item(item: Item):
return item
In this example, the Item
model is defined with attributes that FastAPI validates against the incoming request body. If the request body does not match the Item
model, FastAPI returns a descriptive error response.
3.3 Handling Authentication and Security
Security is paramount in API development. FastAPI provides tools to implement authentication and authorization mechanisms, such as OAuth2 with Password (and hashing), JWT tokens, and API keys.
from fastapi import FastAPI, Depends, HTTPException, status
from fastapi.security import OAuth2PasswordBearer
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
app = FastAPI()
async def get_current_user(token: str = Depends(oauth2_scheme)):
if token != "fake-super-secret-token":
raise HTTPException(
status_code=status.HTTP_401_UNAUTHORIZED,
detail="Invalid authentication credentials",
headers={"WWW-Authenticate": "Bearer"},
)
return {"user": "john doe"}
@app.get("/users/me")
async def read_users_me(current_user: dict = Depends(get_current_user)):
return current_user
The code snippet demonstrates the use of OAuth2PasswordBearer
for token-based authentication. The get_current_user
dependency is invoked before the endpoint logic, ensuring that only authenticated requests are processed.
By mastering these advanced features, developers can build APIs that are not only performant but also maintainable, scalable, and secure. FastAPI's design encourages adherence to best practices and standards, making it an excellent choice for modern API development.
Best Practices and Performance Optimization
4.1 Designing Scalable API Architecture
When constructing an API with FastAPI, scalability must be a foundational consideration. Scalable API architecture ensures that the system can handle growth — in data volume, traffic, or complexity — without compromising performance. To achieve this, one must adhere to stateless design principles, allowing each API call to be independent and capable of being routed to any application instance. This approach facilitates horizontal scaling, where additional instances can be deployed to manage increased load.
Microservices architecture is often employed to enhance scalability. By decomposing an application into smaller, loosely coupled services, developers can isolate and scale high-demand components without affecting the entire system. Each microservice can be encapsulated in a Docker container, orchestrated by Kubernetes for optimal resource utilization and resilience.
Caching strategies further contribute to scalability. Implementing a Redis cache to store frequently accessed data reduces the number of expensive database calls. This not only accelerates response times but also diminishes the load on the database, enabling the API to support more concurrent users.
4.2 Optimizing Response Time and Efficiency
Optimizing an API's response time is critical for user satisfaction and system efficiency. FastAPI's asynchronous request handling is a key feature that improves response times by allowing concurrent processing of requests. This is particularly beneficial for I/O-bound operations, such as database interactions or calls to external services, where the server can handle other tasks while awaiting responses.
Database query optimization is another crucial aspect. Indexing appropriate columns can lead to significant reductions in query execution time. Additionally, using an Object-Relational Mapping (ORM) tool like SQLAlchemy enables developers to write database-agnostic code and optimize queries without delving into SQL intricacies.
Profiling tools can identify bottlenecks in the API. Python's built-in cProfile
module, for instance, can be used to measure the performance of individual functions. By analyzing the profiling data, developers can refactor inefficient code and prioritize optimizations that yield the most significant impact on performance.
In conclusion, building APIs with FastAPI requires a strategic approach to scalability and efficiency. By designing stateless, microservices-based architectures, employing caching, leveraging asynchronous processing, and optimizing database interactions, developers can create robust APIs that stand the test of time and load.
Deployment and Monitoring
5.1 Deploying FastAPI Applications
Deploying FastAPI applications involves several critical steps to ensure that the API is accessible, scalable, and secure. The deployment process typically begins with the selection of a suitable hosting environment, which may range from cloud providers like AWS, Azure, or GCP to container orchestration platforms such as Kubernetes. The use of containers, particularly with Docker, is highly recommended due to the isolation, reproducibility, and scalability they offer.
Once the hosting environment is chosen, the application must be configured to run with a production-grade server. Uvicorn, an ASGI server, is commonly used to serve FastAPI applications, but in a production setting, it should be paired with a server manager like Gunicorn to manage multiple worker processes. This combination allows for handling increased traffic by distributing requests across workers.
To deploy a FastAPI application using Uvicorn with Gunicorn, the following command can be used:
gunicorn -w 4 -k uvicorn.workers.UvicornWorker myapp:app
In this command, -w 4
specifies the number of worker processes, and myapp:app
refers to the FastAPI application object. It is essential to adjust the number of workers based on the available resources and expected workload.
5.2 Monitoring and Logging Best Practices
Effective monitoring and logging are paramount for maintaining the reliability and performance of FastAPI applications. Monitoring involves tracking the application's health, performance metrics, and usage patterns, while logging is concerned with recording events and errors that occur during the application's operation.
Best practices for monitoring include setting up automated alerts based on specific metrics such as response times, error rates, and system resource utilization. Tools like Prometheus for metric collection and Grafana for visualization are often used in conjunction with FastAPI to create comprehensive monitoring solutions.
For logging, it is advisable to implement structured logging, which produces logs in a consistent and machine-readable format, such as JSON. Structured logs make it easier to search and analyze log data, which is crucial for troubleshooting issues. Python's built-in logging module can be configured to output structured logs, and integration with external logging services or platforms can further enhance log management capabilities.
Incorporating these deployment and monitoring strategies ensures that FastAPI applications are robust, performant, and maintainable in a production environment.