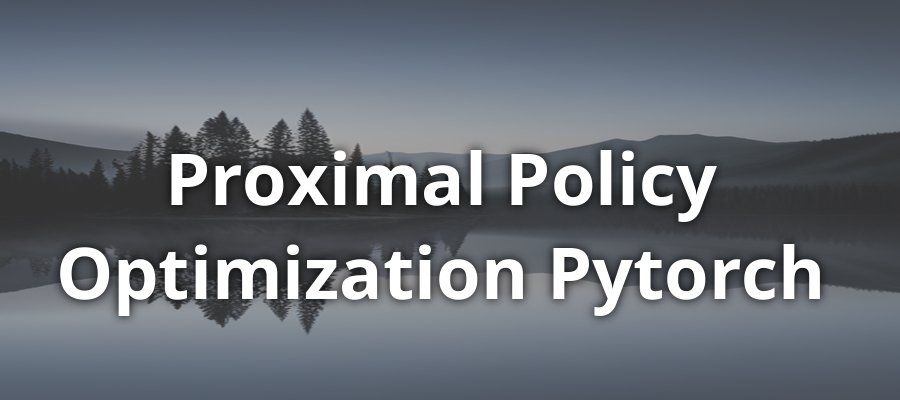
Mastering Proximal Policy Optimization with PyTorch: A Comprehensive Guide
• January 12, 2024
Learn how to implement and optimize Proximal Policy Optimization (PPO) in PyTorch with this comprehensive tutorial. Dive deep into the algorithm and gain a thorough understanding of its implementation for reinforcement learning.
Understanding Proximal Policy Optimization
1.1 Theoretical Foundations of PPO
Proximal Policy Optimization (PPO) is a policy gradient method for reinforcement learning that addresses the limitations of previous algorithms like Trust Region Policy Optimization (TRPO). PPO aims to balance the trade-off between exploration and exploitation by optimizing policy updates, ensuring they are neither too large—risking performance collapse—nor too small—hindering learning progress. The core idea behind PPO is to maintain a stable policy iteration by employing a clipped surrogate objective function, which prevents the policy from deviating excessively from the previous iteration.
1.2 PPO Algorithm Explained
The PPO algorithm iteratively updates policies by maximizing a clipped surrogate objective function. This function uses the probability ratio between the new and old policies, clipped to remove incentives for moving too far from the old policy. The clipping mechanism is defined by a hyperparameter, ε, which dictates the threshold for the ratio. The objective function is typically maximized using stochastic gradient descent (SGD) with multiple epochs over the sampled data to refine the policy parameters.
ratio = new_policy_probs / old_policy_probs
clipped_ratio = torch.clamp(ratio, 1 - ε, 1 + ε)
objective = torch.min(ratio * advantages, clipped_ratio * advantages)
1.3 Advantages of PPO in Reinforcement Learning
PPO offers several advantages over its predecessors in reinforcement learning. Its simplicity in implementation, without the need for complex second-order optimization methods, makes it accessible and computationally efficient. PPO's ability to work with both discrete and continuous action spaces broadens its applicability across various domains. Furthermore, the algorithm's robustness and stability in training have been empirically validated, often resulting in superior performance in terms of sample efficiency and final policy quality.
Implementing PPO with PyTorch
2.1 Setting Up the PyTorch Environment
To begin implementing Proximal Policy Optimization (PPO) using PyTorch, one must first establish a suitable development environment. This involves the installation of PyTorch, a leading deep learning library that provides a flexible platform for building and training neural networks. The environment setup also requires the installation of additional dependencies such as gym
for reinforcement learning tasks, numpy
for numerical operations, and potentially matplotlib
for visualization.
The following code snippet illustrates the installation process for the required packages using pip
, Python's package installer:
pip install torch torchvision torchaudio
pip install gym
pip install numpy
pip install matplotlib
Once the environment is configured, the next step is to import the necessary modules in your Python script:
import torch
import gym
import numpy as np
import matplotlib.pyplot as plt
With the environment ready, developers can proceed to instantiate the reinforcement learning environment and set the stage for the PPO algorithm's implementation.
2.2 Building Actor-Critic Models
The core of the PPO algorithm lies in its actor-critic architecture, which consists of two neural networks: the actor, which determines the policy by mapping states to actions, and the critic, which evaluates the taken actions by estimating the value function. In PyTorch, these models are defined as subclasses of torch.nn.Module
.
Below is an example of a simple actor-critic model structure using PyTorch:
import torch.nn as nn
import torch.nn.functional as F
class ActorCritic(nn.Module):
def __init__(self, state_dim, action_dim):
super(ActorCritic, self).__init__()
self.actor = nn.Sequential(
nn.Linear(state_dim, 64),
nn.ReLU(),
nn.Linear(64, action_dim),
nn.Softmax(dim=-1)
)
self.critic = nn.Sequential(
nn.Linear(state_dim, 64),
nn.ReLU(),
nn.Linear(64, 1)
)
def forward(self, state):
action_probs = self.actor(state)
state_value = self.critic(state)
return action_probs, state_value
This model can be instantiated and used to generate action probabilities and state value estimates given an input state.
2.3 Training and Testing PPO Models
Training the PPO model involves interaction with the environment, collecting data, and optimizing the policy and value function. The training loop typically includes sampling actions using the actor network, evaluating rewards and state values, and performing backpropagation to update the model weights.
A simplified training loop might look like this:
def train_ppo(env, actor_critic, optimizer, num_epochs):
for epoch in range(num_epochs):
state = env.reset()
while True:
# Convert state to tensor
state_tensor = torch.from_numpy(state).float()
# Get action probabilities and state value from the model
action_probs, state_value = actor_critic(state_tensor)
# Sample action from the distribution
action = torch.multinomial(action_probs, 1).item()
# Take action in the environment
next_state, reward, done, _ = env.step(action)
# Calculate loss and perform backpropagation
loss = compute_ppo_loss(action_probs, state_value, reward, done)
optimizer.zero_grad()
loss.backward()
optimizer.step()
if done:
break
state = next_state
Testing the model involves running the trained policy in the environment without performing any learning updates. The goal is to evaluate the performance of the policy:
def test_ppo(env, actor_critic):
state = env.reset()
total_reward = 0
while True:
state_tensor = torch.from_numpy(state).float()
with torch.no_grad():
action_probs, _ = actor_critic(state_tensor)
action = torch.multinomial(action_probs, 1).item()
state, reward, done, _ = env.step(action)
total_reward += reward
if done:
break
return total_reward
The train_ppo
and test_ppo
functions encapsulate the essence of the PPO training and testing processes, respectively. The actual implementation would include additional details such as clipping the policy objective, entropy regularization, and early stopping based on KL divergence.
Optimizing PPO Performance
Optimizing the performance of Proximal Policy Optimization (PPO) algorithms is crucial for achieving high-quality results in reinforcement learning tasks. This section delves into strategies for enhancing PPO's effectiveness, focusing on hyperparameter tuning, input normalization, and performance benchmarking.
3.1 Hyperparameter Tuning Strategies
Hyperparameter tuning is an iterative process aimed at finding the optimal set of parameters that govern the learning process of PPO. Key hyperparameters include learning rate, discount factor, and the number of steps collected per update. A systematic approach to tuning involves grid search, random search, or Bayesian optimization methods. The learning rate dictates the magnitude of updates to the policy network and typically requires a balance to avoid both slow convergence and instability. The discount factor influences the agent's foresight, with higher values placing more emphasis on future rewards. Steps per update affect the trade-off between policy update frequency and the quality of policy estimation. Empirical evaluation suggests starting with values established in literature and incrementally adjusting based on the specific environment and task requirements.
3.2 Normalization Techniques for State Inputs
Normalization of state inputs is a preprocessing step that can significantly impact the learning dynamics of PPO. Normalizing inputs to have zero mean and unit variance can accelerate learning by providing a consistent scale for the input features. This consistency aids the optimization process, as it ensures that gradient updates are not disproportionately affected by the scale of different inputs. Techniques such as batch normalization or layer normalization can be applied, although care must be taken to apply these methods appropriately in the context of reinforcement learning, where data distribution can shift over time.
3.3 Benchmarking and Analysis
Benchmarking PPO performance involves systematic evaluation against a set of predefined metrics. Common metrics include average return, sample efficiency, and stability of learning. Analysis of these metrics allows for the identification of performance bottlenecks and areas for improvement. It is also essential to compare PPO's performance against baseline algorithms to contextualize its effectiveness. Visualization tools such as learning curves and performance heatmaps can aid in interpreting results. Additionally, ablation studies, where components of the algorithm are selectively removed or altered, can provide insights into the contribution of each component to overall performance.
Advanced Topics in PPO
4.1 Multi-Task and Multi-Agent Learning with PPO
Proximal Policy Optimization (PPO) has been established as a robust and versatile algorithm in the realm of reinforcement learning. When considering multi-task learning, PPO's ability to handle multiple objectives simultaneously is of particular interest. In multi-task settings, a single agent learns policies for a variety of tasks, which can lead to more generalized learning and efficient transfer of knowledge between tasks. PPO's objective function can be adapted to accommodate the nuances of multi-task learning by aggregating the expected returns across all tasks, ensuring that the policy improves in a balanced manner.
In the context of multi-agent systems, PPO's scalability and stability are beneficial. Each agent, operating under a shared environment, can utilize PPO to optimize its policy while accounting for the actions of other agents. This is particularly relevant in cooperative scenarios where agents must work together to achieve common goals. PPO's clipped surrogate objective function can be extended to multi-agent scenarios, where the collective reward is maximized while preventing policy updates from diverging significantly from the current policy, thus maintaining stability in the learning process.
4.2 Exploration vs. Exploitation in PPO
The exploration-exploitation trade-off is a fundamental challenge in reinforcement learning. PPO addresses this by using stochastic policy gradients to encourage exploration while the clipping mechanism in the objective function prevents excessive exploitation of the current policy. This balance allows PPO to explore the action space effectively without compromising the stability of policy updates.
Moreover, PPO's use of multiple epochs of stochastic gradient ascent for each batch of data collected encourages a more thorough exploration of the policy space within each update. This iterative refinement process helps in discovering and exploiting high-reward strategies while still maintaining the flexibility to explore when necessary. The entropy bonus, often included in the PPO objective, further promotes exploration by incentivizing the policy to maintain a level of randomness in action selection.
4.3 PPO in Complex Environments
PPO's robustness is tested in complex environments, which are characterized by high-dimensional state and action spaces, partial observability, and intricate reward structures. In such environments, PPO's model-free nature and ability to handle long-term dependencies through the use of advantage estimation are crucial. The algorithm's resilience to hyperparameter changes is also advantageous in these settings, where the optimal configuration may be difficult to determine a priori.
Complex environments often require sophisticated neural network architectures to approximate the policy and value functions. PPO's compatibility with various network architectures, including convolutional and recurrent neural networks, allows it to be applied to a wide range of problems, from playing video games at a superhuman level to controlling robotic systems with high degrees of freedom. The algorithm's sample efficiency and stability during training make it a suitable choice for tasks where data collection is expensive or risky.
In summary, PPO's flexibility and stability make it a strong candidate for tackling advanced topics in reinforcement learning, such as multi-task and multi-agent learning, as well as operating in complex environments. Its ability to balance exploration and exploitation, adapt to various network architectures, and handle intricate reward dynamics underscores its utility in pushing the boundaries of what is achievable with modern reinforcement learning techniques.
Practical Applications and Case Studies
5.1 Real-World Implementations of PPO
Proximal Policy Optimization (PPO) has been successfully applied in various domains, demonstrating its versatility and robustness. In the realm of gaming, PPO has been utilized to train agents that perform at superhuman levels in complex environments. For instance, OpenAI's Dota 2 bots, which are capable of defeating professional human players, leverage PPO for decision-making processes. Beyond gaming, PPO's application extends to robotics, where it aids in the development of autonomous systems capable of navigating and manipulating objects in dynamic environments. These implementations underscore PPO's efficacy in handling high-dimensional state and action spaces.
5.2 Case Study: PPO in Robotics
Robotics presents a challenging domain for reinforcement learning due to the need for real-time decision-making and physical interaction with the world. PPO has emerged as a prominent algorithm in this field, facilitating the training of robots in simulation before transferring learned behaviors to real-world scenarios. A notable example is the deployment of PPO-trained policies in robotic arms for precise object manipulation tasks. The algorithm's ability to learn complex control policies without requiring extensive hand-engineering positions it as a valuable tool for advancing robotic autonomy.
5.3 Case Study: PPO in Finance
In the finance sector, PPO has been explored as a mechanism for algorithmic trading, where the goal is to maximize investment returns while managing risk. By modeling the trading environment as a reinforcement learning problem, PPO can be employed to develop trading strategies that adapt to market conditions. The algorithm's stability and sample efficiency make it suitable for financial applications where data can be scarce and costly. PPO's success in finance illustrates its potential to inform decision-making in stochastic environments with significant economic implications.