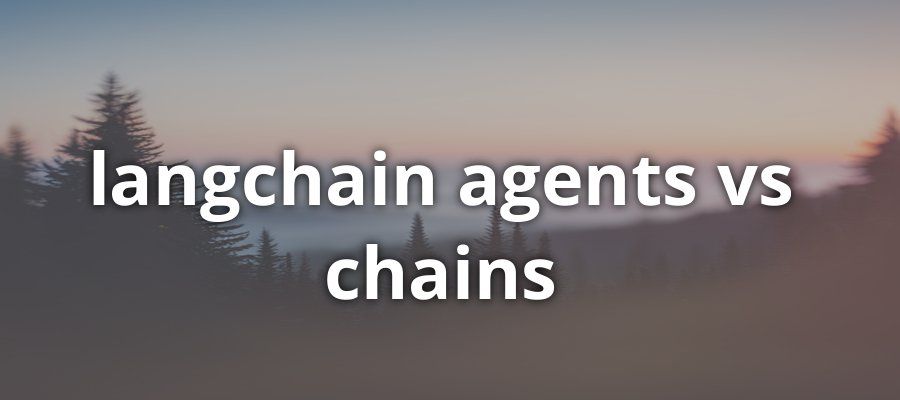
LangChain Agents vs Chains: Understanding the Key Differences
• January 22, 2024
Explore the fundamental disparities between LangChain agents and chains, and how they impact decision-making and process structuring within the LangChain framework. Gain insights into the adaptability of agents and the predetermined nature of chains.
Understanding LangChain: Agents and Chains
1.1 The Basics of LangChain Agents
LangChain agents are autonomous entities within the LangChain framework designed to exhibit decision-making capabilities and adaptability. These agents are constructed to handle complex control flows and are integral to applications requiring dynamic responses. An agent's architecture allows it to process input, engage in decision-making, and execute actions based on a set of defined parameters and learned experiences.
For instance, consider an agent tasked with customer service. It would receive a query, interpret the request, and determine the most appropriate course of action, whether that be providing an answer directly, fetching information from a database, or escalating the issue to a human representative.
1.2 Exploring the Functionality of Chains
Chains in LangChain represent a structured sequence of operations, each with a defined role within a larger process. They are best suited for workflows that are static and do not require deviation from a predetermined path. A chain is composed of multiple links, each representing an operation or a call to a language model, and is executed in a specific order to achieve a desired outcome.
For example, a chain could involve data retrieval from a database, followed by processing through a language model, and culminating in the delivery of a formatted report. The rigidity of chains ensures consistency and reliability in repetitive tasks.
1.3 Comparative Analysis: Agents vs Chains
When comparing agents and chains within the LangChain framework, the key differentiator lies in their approach to task execution. Agents are dynamic, capable of learning and adapting to new scenarios, making them suitable for complex, non-linear workflows. They thrive in environments where decision-making and flexibility are paramount.
Chains, on the other hand, excel in environments where a fixed sequence of steps is required. They provide a reliable method for executing repetitive tasks where variability is neither needed nor desired. The choice between using an agent or a chain depends on the nature of the task at hand and the level of complexity involved.
2. Implementing LangChain Agents
2.1 Designing Autonomous Agents
Autonomous agents within the LangChain framework are designed to operate independently, making decisions and executing actions without external input once deployed. The architecture of these agents is critical, as it must incorporate mechanisms for perception, decision-making, and action execution. To design an autonomous agent, developers must first define the agent's goals and the environment it will interact with. Subsequently, the agent's decision-making model is crafted, often utilizing decision trees, rule-based systems, or machine learning algorithms. Finally, the agent is equipped with a set of actions it can perform, tailored to the tasks it is expected to complete.
2.2 Building Conversational Retrieval Agents
Conversational retrieval agents are specialized LangChain agents that interact with users through natural language to retrieve and provide information. These agents are constructed using a combination of natural language processing (NLP) techniques and retrieval mechanisms. The process begins with the development of a robust language understanding model that can interpret user queries. Following this, a retrieval system is integrated, capable of sourcing the required information from databases, APIs, or other data repositories. The agent then formats this information into a coherent response, maintaining the conversational context.
2.3 Agent Types and Their Use Cases
LangChain supports various agent types, each suited to different use cases. Action Agents, for instance, are designed for discrete tasks, executing one action at a time based on the current context. Plan-and-Execute Agents, in contrast, formulate a series of actions before execution, making them suitable for complex tasks with long-term objectives. The choice between agent types depends on factors such as task complexity, required response time, and the need for adaptability. Understanding the strengths and limitations of each agent type is crucial for developers to effectively implement LangChain agents in their applications.
3. Developing with LangChain Chains
3.1 Chain Construction and Execution
LangChain facilitates the creation of complex workflows through the concept of chains. A chain is a sequence of operations, each encapsulating a discrete computational task. These tasks can range from data retrieval and processing to interaction with language models and external APIs. The construction of a chain involves defining these tasks and the order in which they should be executed.
To instantiate a chain, developers typically employ the LangChain Expression Language (LCEL) or use predefined constructors. The following code snippet demonstrates the instantiation of a basic chain using LCEL:
from langchain.chains import LCELChain
# Define the sequence of operations
operations = [
{"operation": "input", "args": {"prompt": "Enter your query:"}},
{"operation": "llm", "args": {"model_name": "gpt-3"}},
{"operation": "output", "args": {"format": "text"}}
]
# Create the chain
chain = LCELChain(operations)
Execution of a chain is a linear process where the output of one operation becomes the input to the next. This ensures a clear and maintainable flow of data through the chain.
3.2 Integrating Tools with Chains
LangChain's architecture allows for the seamless integration of various tools within a chain. These tools can be language models, databases, or any service that exposes an API. Integration is achieved by defining operations within the chain that interact with these tools.
For example, integrating a database retrieval operation within a chain can be accomplished as follows:
from langchain.chains import LCELChain
# Define the sequence of operations, including a database retrieval
operations = [
{"operation": "input", "args": {"prompt": "Enter your query:"}},
{"operation": "database_retrieval", "args": {"query": "SELECT * FROM table WHERE condition"}},
{"operation": "llm", "args": {"model_name": "gpt-3"}},
{"operation": "output", "args": {"format": "text"}}
]
# Create the chain with the integrated database retrieval
chain = LCELChain(operations)
This integration capability enables developers to construct chains that are not only powerful but also highly adaptable to various use cases.
3.3 When to Opt for Chains Over Agents
Choosing between chains and agents depends on the nature of the task at hand. Chains are ideal for tasks with a well-defined sequence of steps that do not require deviation from the established process. They provide a straightforward, repeatable approach to handling data and operations.
In contrast, agents are better suited for scenarios that demand decision-making, adaptability, and complex control flows. Agents can evaluate the context, make decisions, and dynamically alter their behavior, which is beyond the scope of a static chain.
The decision to use chains over agents should be guided by the requirement for either a structured, predictable process or a dynamic, context-aware system.
Advanced Techniques in LangChain
4.1 Optimizing Performance with LangChain Agents
LangChain agents are designed to facilitate complex interactions with large language models (LLMs). Performance optimization of these agents is critical for efficient operation. One approach to optimization is the refinement of input prompts. By crafting precise prompts that align closely with the LLM's training data, one can reduce the computational overhead and improve response accuracy.
Another aspect of optimization involves the management of state. Agents that maintain a contextual state can deliver more coherent and contextually relevant responses. This requires careful design to ensure that the state is updated appropriately with each interaction, balancing the computational load with the need for nuanced understanding.
Lastly, the use of caching mechanisms can significantly enhance performance. By storing frequently accessed information or responses, agents can quickly retrieve data without redundant processing. Implementing a smart caching strategy that anticipates user needs can lead to substantial improvements in speed and efficiency.
4.2 Enhancing Capabilities with Chain Extensions
Chain extensions in LangChain offer a modular approach to expanding the capabilities of LLMs. These extensions can be thought of as middleware, intercepting and processing data between the LLM and the end-user. For instance, a chain extension could be designed to perform sentiment analysis on generated text, providing additional layers of insight.
Another application of chain extensions is in the realm of data transformation. Extensions can be used to format LLM outputs into structured data, making it easier to integrate with other systems or databases. This is particularly useful for tasks that require a high degree of data uniformity, such as reporting or analytics.
Furthermore, chain extensions can be leveraged to implement custom business logic. By inserting domain-specific rules and processes into the chain, one can tailor the behavior of the LLM to meet specific organizational needs. This customization is key to deploying LLMs in specialized fields where generic responses are insufficient.
In summary, chain extensions are a powerful tool for enhancing the functionality of LangChain agents, enabling them to serve more sophisticated and specialized purposes.
LangChain in Practice: Case Studies
5.1 Real-World Applications of Agents
LangChain agents are deployed in various domains to enhance decision-making and adaptability. For instance, in customer service, agents interpret user queries and retrieve relevant information, streamlining support. In finance, they analyze market data to provide investment insights, demonstrating their utility in data-rich environments. These applications underscore agents' capacity to handle dynamic scenarios and complex decision trees, making them indispensable for tasks necessitating cognitive flexibility.
5.2 Innovative Uses of Chains in Industry
Chains, with their sequential and fixed-step nature, are leveraged in industries where workflow standardization is critical. In manufacturing, chains ensure quality control by systematically checking each production stage. In software development, continuous integration and deployment pipelines are structured as chains, automating code testing and deployment. These use cases highlight chains' effectiveness in scenarios with well-defined processes and the need for consistency and repeatability.