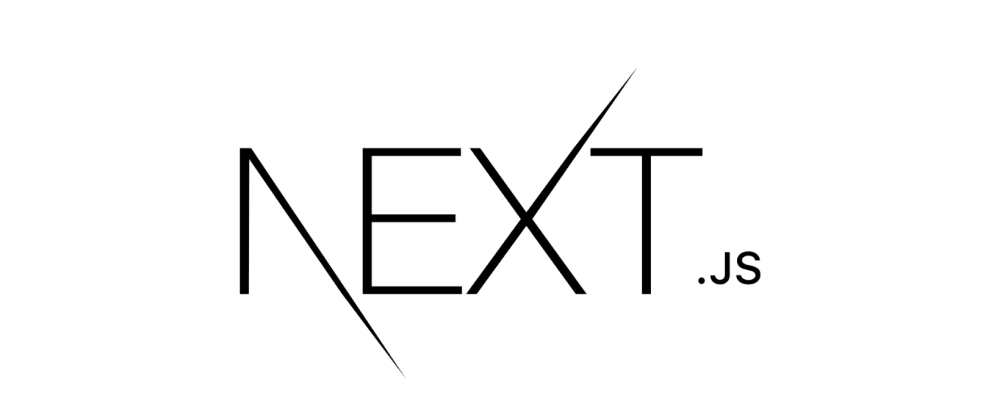
Next.js Cron Jobs with AWS Lambda and TypeScript
• September 27, 2023
Explore how to efficiently set up cron jobs using AWS Lambda in TypeScript. Learn why Next.js isn't ideal for cron tasks and how AWS Lambda provides a robust, scalable solution
Table of Contents
- Introduction to AWS Lambda and Cron Jobs
- Why Not Use Next.js for Cron Jobs?
- Advantages of Using AWS Lambda for Cron Jobs
- Setting Up Your Environment
- Writing a Cron Job with AWS Lambda in TypeScript
- Deploying Your Cron Job
- Final Thoughts
1. Introduction to AWS Lambda and Cron Jobs
If you've stumbled upon this article, chances are you're familiar with the modern web framework, Next.js. However, while Next.js is fantastic for building user interfaces, when it comes to running scheduled tasks or cron jobs, we need to think outside the box. One such solution is AWS Lambda. Today, I'll guide you through setting up cron jobs using AWS Lambda, specifically using TypeScript.
2. Why Not Use Next.js for Cron Jobs?
Next.js is primarily designed for SSR (Server Side Rendering) and static site generation. While you could potentially set up cron jobs using Node.js with your Next.js app, you'd encounter a few hurdles:
- Scalability: Handling multiple users or tasks might become cumbersome, requiring manual scaling.
- Reliability: The risk of your Next.js application crashing increases with added background tasks, impacting the user experience.
- Separation of Concerns: Intertwining background tasks with user-facing logic makes maintenance difficult.
3. Advantages of Using AWS Lambda for Cron Jobs
Scalability: AWS Lambda automatically scales, handling each trigger individually.
Cost-Efficiency: You pay only for the compute time you consume. If your cron job runs for a short period, you pay for just that duration.
Maintenance: AWS provides monitoring tools like CloudWatch to monitor the execution of your Lambda functions.
Simplicity: Without the need for any server setup, you can focus on your code.
4. Setting Up Your Environment
Before diving in, ensure you've:
- An AWS account set up.
- AWS CLI installed and configured.
- Node.js and TypeScript set up in your development environment.
5. Writing a Cron Job with AWS Lambda in TypeScript
import { APIGatewayProxyHandler } from 'aws-lambda';
import 'source-map-support/register';
export const hello: APIGatewayProxyHandler = async (event, _context) => {
// Your cron logic here
return {
statusCode: 200,
body: JSON.stringify({
message: 'Cron job executed successfully!',
}),
};
};
To set up a cron schedule for your Lambda:
- Navigate to AWS Management Console.
- Go to
Lambda
>Functions
>Create function
. - Name your function, choose the Node.js runtime, and define the handler.
- In the designer, click on
Add trigger
, and chooseEventBridge (CloudWatch Events)
. - Configure your cron or rate expression. For instance,
cron(0 12 * * ? *)
runs your Lambda every day at noon.
6. Deploying Your Cron Job
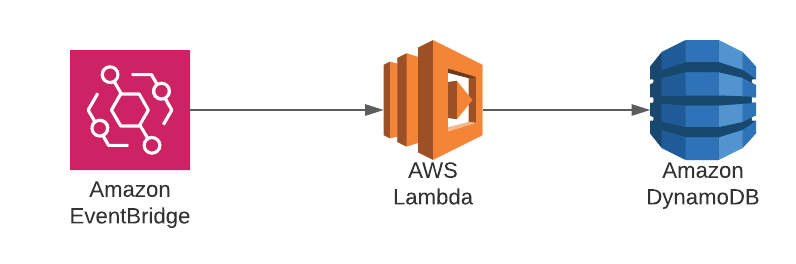
For deployment:
- Bundle your TypeScript code and its dependencies.
- Upload your ZIP bundle to AWS Lambda.
Here's a basic script to aid deployment:
tsc
zip -r function.zip .
aws lambda update-function-code --function-name your-lambda-name --zip-file fileb://function.zip
7. Final Thoughts
Using AWS Lambda for cron jobs offers a plethora of advantages, from scalability to simplicity. Though Next.js is a powerful framework for UI rendering, AWS Lambda shines when it comes to backend operations like cron jobs. As you progress, you'll find this separation beneficial for both performance and maintainability.