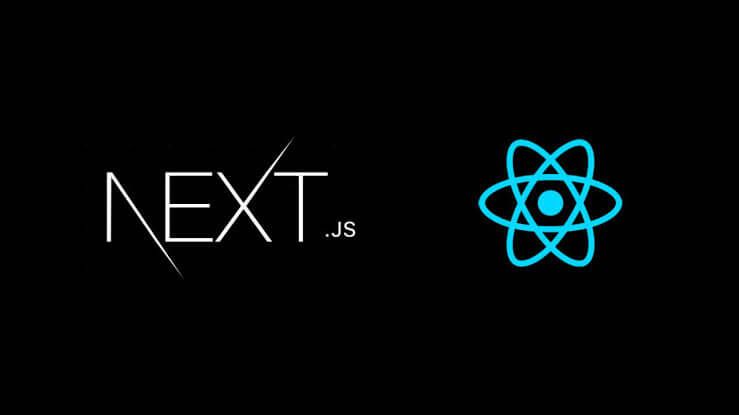
Next.js vs React: A Comprehensive Comparison for Front-End Development
• November 7, 2023
Explore the differences between Next.js and React, including performance, SEO, and developer experience, to determine the best framework for your next project.
Next.js vs React: A Technical Deep Dive for Developers
1. Introduction to Next.js and React
React has become a cornerstone in the world of modern web development, offering a robust framework for building dynamic user interfaces. Developed by Facebook, React's component-based architecture has paved the way for efficient and scalable applications. However, as developers seek to streamline their development process further, Next.js emerges as a powerful tool built on top of React. Next.js is a React framework that provides a set of features, such as server-side rendering and static site generation, aimed at improving the performance and developer experience of web applications.
While React lays the foundation for building interactive user interfaces with its virtual DOM and reactive data flow, Next.js extends these capabilities by offering a structured framework that simplifies common tasks in web development. This includes page routing, image optimization, and API routes, which are essential for building full-fledged applications. The synergy between React's flexibility and Next.js's conventions makes for a compelling combination for developers looking to create high-quality web applications.
2. Understanding the Technical Problem
The primary technical challenge that developers face when using React alone is the need to set up and configure various aspects of a project manually. This includes routing, code splitting, and server-side rendering, which are not provided out-of-the-box by React. As applications grow in complexity, managing these configurations can become cumbersome and time-consuming, leading to potential performance bottlenecks and a steeper learning curve for new developers joining the project.
Next.js addresses these challenges by providing a standardized framework that handles these concerns by default. It offers a file-system-based router, automatic code splitting, and pre-rendering capabilities, which significantly reduce the initial setup and ongoing maintenance required for a React application. By abstracting away these complexities, Next.js allows developers to focus more on the business logic and user experience rather than the boilerplate code and configuration.
3. Proposed Solution
The proposed solution to the challenges faced in React development is to adopt Next.js as the framework of choice for building React applications. Next.js streamlines the development process by providing a suite of built-in features that cater to the needs of modern web applications. It offers a hybrid static and server rendering approach, which allows developers to choose the best rendering method for each page, optimizing for performance and SEO.
Additionally, Next.js comes with built-in support for CSS-in-JS libraries, API routes for building serverless functions, and a plugin ecosystem that further extends its capabilities. By leveraging Next.js, developers can reduce the time spent on configuration and tooling, leading to faster development cycles and a more efficient workflow.
4. Implementation Details
// pages/posts/[id].js
import { useRouter } from 'next/router';
const Post = () => {
const router = useRouter();
const { id } = router.query;
return <p>Post: {id}</p>;
};
export default Post;
When implementing a Next.js application, developers will notice the framework's opinionated structure, which guides the organization of files and directories. Pages are automatically routed based on the file system, and dynamic routes can be easily created by using bracket notation in file names. This convention-over-configuration approach simplifies the routing mechanism, which is often a complex aspect of React applications.
Next.js also provides a getStaticProps
and getServerSideProps
functions for fetching data at build time or request time, respectively. This allows for optimized data fetching strategies that can improve the loading times and interactivity of the application. The framework's focus on performance is evident in its automatic code splitting, which ensures that only the necessary code is loaded for each page, reducing the overall bundle size.
5. Testing and Quality Assurance
Testing is a critical component of any development process, and Next.js applications are no exception. The framework is compatible with popular testing libraries such as Jest and React Testing Library, allowing developers to write unit and integration tests for their components and pages. End-to-end testing can also be performed using tools like Cypress or Playwright, ensuring that the application functions correctly from the user's perspective.
Quality assurance in Next.js projects involves not only testing the application's functionality but also ensuring that best practices are followed for performance and accessibility. Lighthouse and other performance auditing tools can be used to identify areas for improvement, and automated accessibility testing can help maintain a high standard of inclusivity for all users.
6. Performance Optimization
// This example shows the use of next/image for optimized image loading
import Image from 'next/image';
const MyImage = () => (
<Image
src="/me.png" // Route of the image file
height={144} // Desired size with correct aspect ratio
width={144} // Desired size with correct aspect ratio
alt="Your Name"
/>
);
export default MyImage;
Performance optimization is a key consideration in web development, and Next.js provides several features to help developers achieve fast-loading applications. Image optimization is handled automatically with the next/image
component, which resizes and optimizes images on the fly. The framework also supports incremental static regeneration, allowing pages to be updated in the background without requiring a full rebuild of the site.
Code splitting and lazy loading are built into Next.js, ensuring that users only download the code necessary for the page they are visiting. This results in faster initial load times and a more responsive user experience. Developers can further optimize performance by analyzing bundle sizes with tools like Webpack Bundle Analyzer and implementing caching strategies for static assets.
7. Security Considerations
Security is paramount in web application development, and Next.js provides several built-in mechanisms to help safeguard applications. Automatic escaping of user input helps prevent cross-site scripting (XSS) attacks, and the framework's API routes are designed to be secure by default, reducing the risk of server-side vulnerabilities.
Developers should also follow security best practices such as implementing proper authentication and authorization, using secure headers, and keeping dependencies up to date. Next.js's active community and regular updates ensure that security concerns are addressed promptly, providing developers with peace of mind.
8. Future Enhancements
The Next.js framework is continuously evolving, with new features and improvements being added regularly. Future enhancements may include further optimizations for performance, more integrations with third-party services, and enhanced developer tooling. The framework's open-source nature allows the community to contribute to its development, ensuring that it stays at the forefront of web technology trends.
As the ecosystem around Next.js grows, developers can expect to see advancements in areas such as edge computing, modular architecture, and real-time collaboration features. These enhancements will continue to solidify Next.js as a leading solution for building modern, high-performance web applications.
9. Conclusion
Next.js offers a compelling solution for developers looking to build React applications with improved performance, scalability, and developer experience. By addressing common technical challenges and providing a suite of built-in features, Next.js streamlines the development process and allows developers to focus on creating engaging user experiences. As the web development landscape evolves, Next.js is well-positioned to adapt and provide developers with the tools they need to succeed in building cutting-edge applications.
10. References
To further explore the differences between Next.js and React, and to gain a deeper understanding of the technical aspects discussed in this article, the following references are recommended:
- React Documentation: https://reactjs.org/docs/getting-started.html
- Next.js Documentation: https://nextjs.org/docs
- Vercel's Next.js GitHub Repository: https://github.com/vercel/next.js
- Webpack Bundle Analyzer: https://www.npmjs.com/package/webpack-bundle-analyzer
- Lighthouse: https://developers.google.com/web/tools/lighthouse
By leveraging these resources, developers can deepen their knowledge and stay up-to-date with the latest best practices in web development using Next.js and React.