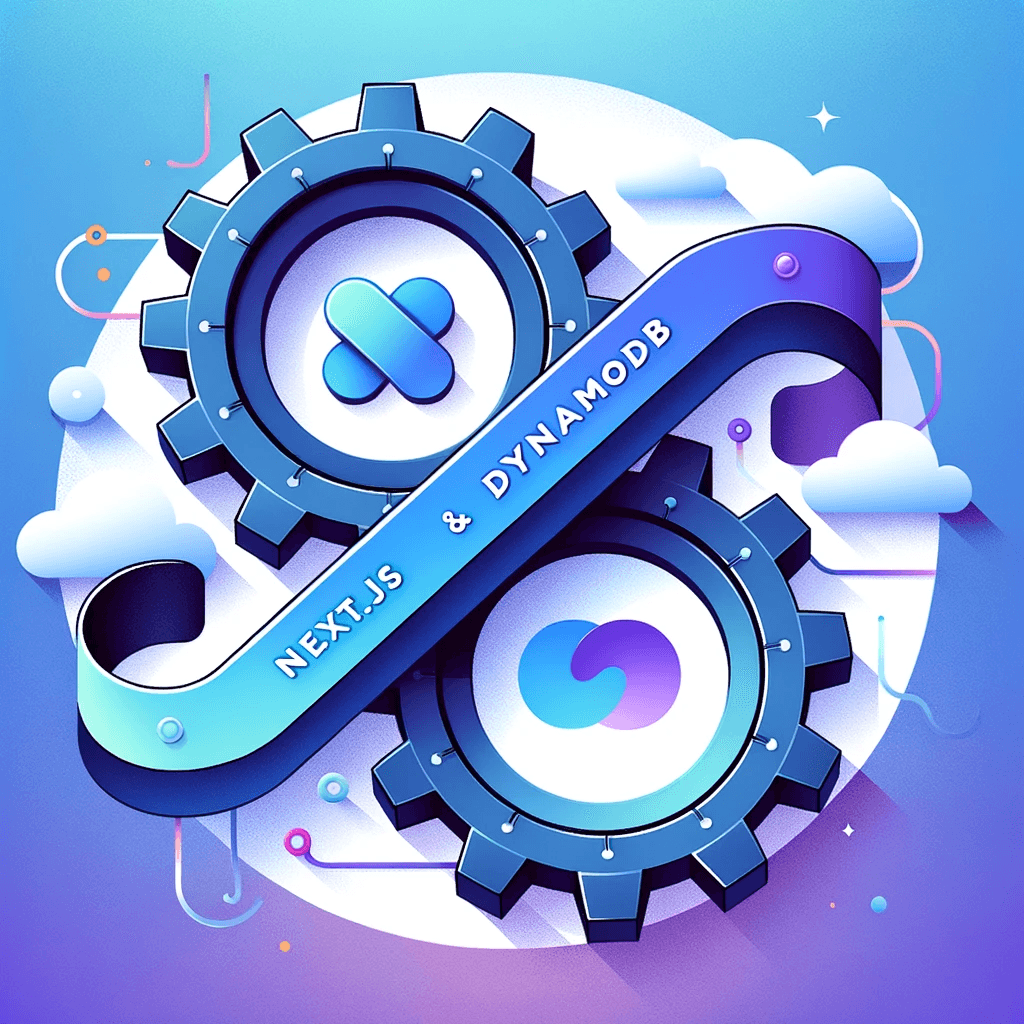
Next-auth with Dynamodb
• October 16, 2023
Explore the power of Next-Auth and AWS DynamoDb to create robust Next.js applications. This comprehensive guide sheds light on integrating AWS DynamoDB for efficient user data storage.
Table of Contents
- Introduction
- Understanding Next.js
- What is Next-Auth?
- High-Level Overview of Next-Auth
- OAuth 2.0 Provider Exchange Process
- Setting Up Next-Auth with GitHub as a Provider
- Breaking Down the Code
- Configuring DynamoDB as the Datastore
- Why DynamoDB?
- Setting Up DynamoDB for Next-Auth
- Final Thoughts
- References
Introduction
In the evolving landscape of web development, the integration of efficient tools and processes is paramount. This guide will delve into the technical intricacies of integrating Next.js with Next-Auth. We will utilize GitHub as the authentication provider and DynamoDB for data storage.
Understanding Next.js
Next.js is a popular React framework that provides features like server-side rendering and static site generation. Its ability to offer a developer-friendly environment without compromising performance has made it a favorite in the development community.
What is Next-Auth?
High-Level Overview of Next-Auth
Next-Auth is a comprehensive authentication solution for Next.js applications. It abstracts away the complexities of user authentication and allows developers to easily integrate various third-party authentication providers.
OAuth 2.0 Provider Exchange Process
OAuth 2.0 is an authorization framework that allows third-party applications to access user data without exposing their passwords. The process involves:
- Requesting an Authorization Code: Your app directs the user to the provider's authorization endpoint.
- User Grants Permission: The user logs in and authorizes your app.
- Getting an Access Token: Your app exchanges the authorization code for an access token.
- Accessing the User's Information: Using the access token, your app can request user information from the provider.
Setting Up Next-Auth with GitHub as a Provider
Before we delve into configuration, it's essential to understand the code structure and the crucial elements of Next-Auth. Here's a snippet to guide our configuration:
import NextAuth, {SessionStrategy} from 'next-auth'
import GithubProvider from 'next-auth/providers/github'
import {DynamoDB, DynamoDBClientConfig} from "@aws-sdk/client-dynamodb";
import {DynamoDBDocument} from "@aws-sdk/lib-dynamodb";
import {DynamoDBAdapter} from "@auth/dynamodb-adapter";
const config: DynamoDBClientConfig = {
region: process.env.NEXT_AUTH_AWS_REGION,
};
const client = DynamoDBDocument.from(new DynamoDB(config), {
marshallOptions: {
convertEmptyValues: true,
removeUndefinedValues: true,
convertClassInstanceToMap: true,
},
})
export const authOptions = {
adapter: DynamoDBAdapter(
client, {
tableName: process.env.NEXT_AUTH_USER_TABLE_NAME!,
}
),
site: process.env.NEXTAUTH_URL,
providers: [GithubProvider({
clientId: process.env.GITHUB_CLIENT_ID!,
clientSecret: process.env.GITHUB_CLIENT_SECRET!,
}),],
debug: true,
callbacks: {
session({session, token}) {
session.user = {
...session.user,
id: token.sub
};
session.token = token;
return session
},
jwt({token, profile, user}) {
if (profile) {
token.image = profile.avatar_url || profile.picture
}
return token
},
},
pages: {
signIn: '/sign-in'
},
session: {
strategy: 'jwt' as SessionStrategy
}
};
const handler = NextAuth(authOptions);
export {handler as GET, handler as POST}
4.1. Breaking Down the Code
Initial Imports: We start by importing necessary modules from next-auth, the GitHub provider from next-auth/providers/github, AWS SDK packages related to DynamoDB, and the DynamoDB adapter for authentication.
DynamoDB Configuration: The config constant sets the AWS region for DynamoDB based on an environment variable. Following that, we create a client for DynamoDB, specifying options for data marshalling.
Auth Options Configuration: The authOptions constant is where we configure various authentication settings:
- adapter: This specifies DynamoDB as our datastore, leveraging the client we just set up.
- site: Points to the base URL of our Next.js application.
- providers: We are using the GitHub provider for authentication, providing the client ID and secret fetched from environment variables.
- debug: This is set to true for debugging purposes.
- callbacks: Contains functions for session and JWT handling.
- pages: Override the default sign-in page.
- session: Specifies JWT as our session strategy. Handler Initialization: The handler leverages the NextAuth function, passing in our authOptions. The handler is then exported to handle both GET and POST requests.
Configuring DynamoDB as the Datastore
5.1. Why DynamoDB?
DynamoDB, AWS's NoSQL database, scales seamlessly with high performance. It's perfect for our user information storage due to its flexibility and scalability.
Final Thoughts With the convergence of Next.js, Next-Auth, DynamoDB, and AWS services, we've forged a powerful toolset for modern web development. Mastering these integrations not only streamlines the development process but also ensures the delivery of high-performance applications.