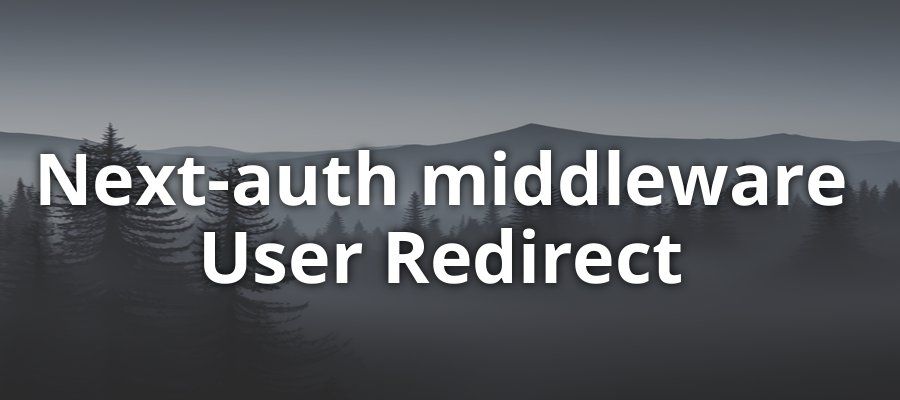
Next-Auth Middleware: Seamless User Redirects
• February 19, 2024
Learn how to effortlessly redirect users with Next-Auth middleware for a smoother, more secure user experience. Perfect for dashboards and private areas.
Introduction to Next-auth Middleware and User Redirects
In the evolving landscape of web development, ensuring secure and efficient user authentication processes is paramount. NextAuth.js emerges as a powerful solution, particularly when integrated with Next.js applications. This section delves into the foundational aspects of NextAuth.js middleware and its pivotal role in managing user redirects within web applications.
1.1 Understanding NextAuth.js Middleware
NextAuth.js is an open-source authentication library designed for Next.js applications, offering developers a seamless authentication experience. It simplifies implementing login systems, supporting various providers such as Google, Facebook, and GitHub, among others. The middleware feature of NextAuth.js, introduced in version 4, enhances its functionality by enabling automatic handling of session and authentication states across pages.
The middleware operates at the server level, intercepting requests before they reach the page. This allows for decisions to be made about whether a user should be allowed access to a page or be redirected based on their authentication status. For example, it can redirect unauthenticated users to a login page when they attempt to access protected routes.
import { withAuth } from 'next-auth/middleware';
import { NextResponse } from 'next/server';
export default withAuth(req => {
if (req.nextUrl.pathname.startsWith('/admin')) {
if (req.nextauth.token.userRole !== 'Admin') {
return NextResponse.redirect(new URL('/dashboard', req.url));
}
}
if (req.nextUrl.pathname.startsWith('/premium')) {
if (req.nextauth.token.userRole !== 'Premium') {
return NextResponse.redirect(new URL('/dashboard', req.url));
}
}
});
export const config = {
matcher: ['/admin/:path*', '/premium/:path*']
};
This snippet demonstrates how NextAuth.js middleware can be utilized to conditionally redirect users based on their roles. It showcases the middleware's ability to inspect the request and make routing decisions accordingly.
1.2 The Role of Middleware in User Authentication and Redirects
Middleware in the context of NextAuth.js serves as a gatekeeper, ensuring that only authenticated users can access specific parts of an application. It plays a crucial role in enhancing security by preventing unauthorized access to protected routes. Furthermore, it contributes to improving the user experience by redirecting users to appropriate pages based on their authentication status.
One of the key benefits of using NextAuth.js middleware is its flexibility. Developers can define custom behavior for redirects, allowing for a tailored authentication flow that meets the specific needs of their application. For instance, middleware can be configured to redirect users to a custom sign-in page instead of the default NextAuth.js sign-in page.
The middleware also supports asynchronous operations, enabling developers to perform additional checks or fetch user roles from a database before deciding on the redirect. This level of control makes NextAuth.js middleware a powerful tool in the developer's arsenal for managing authentication flows.
In summary, NextAuth.js middleware offers a robust solution for handling user authentication and redirects in Next.js applications. Its integration into the Next.js framework simplifies the development of secure and user-friendly web applications, making it an essential tool for modern web developers.# Implementing User Redirects with Next-auth Middleware
In this section, we delve into the practical aspects of implementing user redirects within a Next.js application using NextAuth.js middleware. This functionality is crucial for managing user access and ensuring that users are directed to appropriate content based on their authentication status or role. We will cover the configuration of NextAuth.js to enable secure user redirects and address common issues that may arise during implementation.
Configuring NextAuth.js for Secure User Redirects
To configure NextAuth.js for secure user redirects, it's essential to understand how middleware works in Next.js and how it integrates with NextAuth.js. Middleware allows you to run server-side code before a page is rendered, enabling you to perform checks or modifications to the request.
Here's a step-by-step guide to configuring user redirects:
-
Setup NextAuth.js Middleware: First, ensure that you have NextAuth.js and its middleware correctly set up in your application. You should have a
[...nextauth].js
file in yourpages/api/auth
directory with your authentication providers configured. -
Configure Middleware for Specific Routes: In your
middleware.js
file, use thematcher
property to specify which paths should trigger the middleware. This is where you define the routes that require user authentication.export { default } from "next-auth/middleware"; export const config = { matcher: ["/admin/:path*", "/protected/:path*"] };
-
Implement Redirect Logic: Within the middleware, use the
getToken
function fromnext-auth/jwt
to check if the user has a valid session token. If not, redirect the user to the sign-in page or another specified route.import { getToken } from "next-auth/jwt"; import { NextResponse } from "next/server"; export async function middleware(request) { const token = await getToken({ req: request }); if (!token && process.env.NEXTAUTH_URL) { return NextResponse.redirect(process.env.NEXTAUTH_URL); } }
-
Test Your Configuration: After setting up the middleware, test your application to ensure that unauthenticated users are redirected as expected. It's also important to verify that authenticated users can access protected routes without issues.
Troubleshooting Common Redirect Issues
Despite careful configuration, you may encounter issues with user redirects. Here are some common problems and their solutions:
-
Infinite Redirect Loops: This occurs when the destination of a redirect also triggers a redirect, creating a loop. Ensure that your redirect logic correctly differentiates between authenticated and unauthenticated users to prevent this.
-
Middleware Not Triggering: If your middleware does not seem to be executing, check that it's correctly placed in the
middleware.js
file at the root of your Next.js project. Also, verify that thematcher
configuration matches the paths you intend to protect. -
Token Not Found: If the middleware fails to retrieve the user's session token, ensure that your NextAuth.js configuration is correct and that the session token is being properly set during authentication.
By following these guidelines and troubleshooting tips, you can effectively implement secure user redirects in your Next.js application using NextAuth.js middleware. This not only enhances the security of your application but also improves the user experience by directing users to the appropriate content based on their authentication status.# Advanced Techniques and Best Practices
In this section, we delve into advanced techniques and best practices for utilizing Next-auth middleware in your Next.js applications. These strategies are designed to enhance security, improve user experience, and ensure efficient session handling and token management. By implementing these practices, developers can create more robust and secure web applications.
3.1 Leveraging JWT with Next.js Middleware for Enhanced Security
JSON Web Tokens (JWT) are an open standard (RFC 7519) that define a compact and self-contained way for securely transmitting information between parties as a JSON object. When integrated with Next.js middleware, JWTs offer a powerful method to enhance application security.
Understanding JWT in Next.js Middleware
JWTs can be used in Next.js middleware to authenticate and authorize user requests. This process involves generating a token upon user login and verifying this token in subsequent requests to protect routes or resources. The token typically contains encoded user information and permissions, which the server decodes to determine access rights.
Implementing JWT Authentication
To implement JWT authentication with Next.js middleware, follow these steps:
- Token Generation: Upon successful login, generate a JWT containing the user's information and permissions. Use a secret key to sign the token.
import jwt from 'jsonwebtoken';
const user = { id: 'userId', name: 'userName', role: 'userRole' };
const secret = process.env.JWT_SECRET;
const token = jwt.sign(user, secret, { expiresIn: '1h' });
- Token Verification: Create middleware to verify the token in each protected request. Extract the token from the request headers, decode it, and validate the user's permissions.
import jwt from 'jsonwebtoken';
export const verifyToken = (req, res, next) => {
const token = req.headers.authorization?.split(' ')[1]; // Bearer <token>
if (!token) {
return res.status(403).json({ message: 'A token is required for authentication' });
}
try {
const decoded = jwt.verify(token, process.env.JWT_SECRET);
req.user = decoded;
} catch (err) {
return res.status(401).json({ message: 'Invalid Token' });
}
return next();
};
- Middleware Integration: Apply the verification middleware to protect routes or specific API endpoints.
import express from 'express';
import { verifyToken } from './middleware/auth';
const app = express();
app.get('/protected-route', verifyToken, (req, res) => {
res.send('This route is protected');
});
Best Practices for JWT with Next.js Middleware
- Secure Token Storage: Store JWTs securely on the client side, preferably in HTTPOnly cookies, to prevent XSS attacks.
- Token Expiration: Implement token expiration and refresh tokens to limit the duration of a potential attack.
- Environment Variables: Store secret keys and sensitive information in environment variables, not in your codebase.
3.2 Optimizing Session Handling and Token Management
Efficient session handling and token management are crucial for maintaining a secure and user-friendly web application. Next-auth provides built-in support for session management, but there are practices you can adopt to optimize this process.
Session Management in Next-auth
Next-auth automatically handles sessions, creating a session token upon login and destroying it on logout. However, developers can customize session behavior to suit their application needs.
Customizing Session Settings
To customize session settings in Next-auth, modify the session
and jwt
callbacks in your Next-auth configuration.
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
],
callbacks: {
async jwt(token, user) {
if (user) {
token.id = user.id;
}
return token;
},
async session(session, token) {
session.user.id = token.id;
return session;
},
},
});
Token Refresh Strategy
Implement a token refresh strategy to renew sessions without requiring the user to log in again. This can be achieved by using refresh tokens or custom logic to renew the session before it expires.
Best Practices for Session and Token Management
- Regularly Rotate Secrets: Regularly change secret keys used for signing tokens to mitigate the impact of a compromised key.
- Monitor Session Activity: Implement monitoring to detect unusual session patterns that may indicate unauthorized access.
- Secure Cookies: Use secure, HTTPOnly, and SameSite cookies for storing tokens to prevent CSRF and XSS attacks.
By following these advanced techniques and best practices, developers can leverage Next-auth middleware to create secure, efficient, and user-friendly Next.js applications.