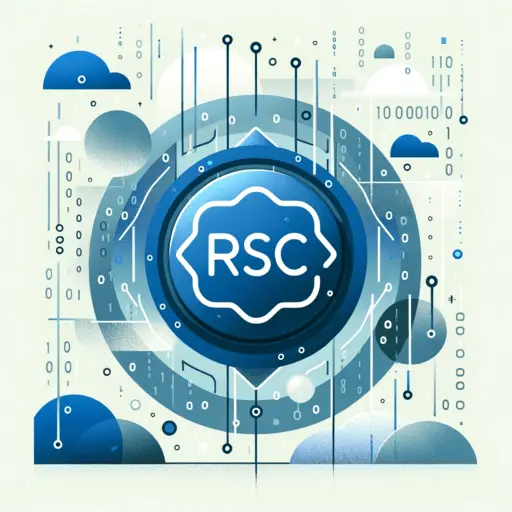
Mastering React Server Components: A Comprehensive Guide
• November 7, 2023
Dive deep into the world of React Server Components and learn how to leverage their power to improve performance and simplify complex paradigms in your applications.
Understanding React Server Components
1. Introduction to React Server Components
React has been a game-changer in the world of web development since its inception, offering a declarative, efficient, and flexible way to build user interfaces. As the library evolves, it introduces new paradigms that aim to enhance performance and developer experience. One such innovation is React Server Components (RSC), which marks a significant shift in how components can be rendered and delivered to the client. This article delves into the technicalities of React Server Components, providing developers with a comprehensive understanding of this powerful feature.
React Server Components offer a new method of rendering that allows developers to write components that render exclusively on the server. This approach differs from traditional client-side rendering and even from the well-known server-side rendering (SSR). By leveraging RSC, developers can optimize their applications by reducing the amount of code shipped to the client, thus improving load times and overall performance.
2. Understanding Server Side Rendering (SSR)
Server Side Rendering (SSR) is a technique used in modern web development where the initial rendering of a web page is performed on the server rather than in the browser. This process results in a fully rendered page being sent to the client, which can be immediately displayed. SSR is particularly beneficial for improving the performance of web applications, as it can significantly reduce the time to first paint and enhance SEO by allowing search engines to crawl content more effectively.
However, SSR also comes with its own set of challenges. The server has to rebuild the entire page on every request, which can lead to increased server load and potentially slower response times for dynamic content. Additionally, once the page is delivered to the client, the browser still needs to download, parse, and execute the JavaScript to become fully interactive, which can add to the perceived load time for users.
3. Differentiating React Server Components from Server-Side Rendering (SSR)
React Server Components (RSC) are often confused with Server-Side Rendering (SSR), but they serve different purposes and operate under distinct paradigms. While SSR is about sending a fully rendered page to the client, RSC focuses on rendering certain components on the server, which do not include any client-side interactivity. These components are then serialized and sent to the client, where they can be hydrated if necessary.
The key difference lies in the fact that RSC allows developers to split their codebase into server and client components. Server components have the ability to access server-side resources, databases, and file systems directly, which is not possible with SSR. This separation of concerns leads to a more efficient loading strategy, as only the necessary client-side code is sent to the browser, reducing the overall bundle size.
4. Advantages of Rendering on the Server
Rendering on the server provides several advantages that can greatly enhance the performance and user experience of web applications. One of the primary benefits is the reduction in the amount of JavaScript that needs to be sent to the client. Since server components handle much of the heavy lifting, the client receives a lighter payload, which translates to faster load times and a more responsive application.
Another advantage is the improved SEO, as search engines can index the content more effectively when it's rendered on the server. This is because the rendered HTML is immediately available, making it easier for search engine bots to crawl and understand the content of the page. Additionally, server rendering can provide a better experience for users on slow connections or with limited processing power, as the initial render is completed on the server, requiring less work from the client's device.
5. The Concept of Client Boundaries
// Message.server.js
import React from 'react';
// This is a server component that fetches data and renders it.
function Message({ id }) {
// Fetch data from the server-side database or filesystem
const message = fetchMessageById(id); // Hypothetical function to fetch data
return <div>{message.content}</div>;
}
export default Message;
// InteractiveButton.client.js
import React from 'react';
// This is a client component that handles interactivity.
function InteractiveButton() {
const [clicked, setClicked] = React.useState(false);
const handleClick = () => {
setClicked(true);
};
return (
<button onClick={handleClick}>
{clicked ? 'Clicked!' : 'Click me'}
</button>
);
}
export default InteractiveButton;
Client boundaries are a fundamental concept in React Server Components that define the separation between components that are rendered on the server and those that are rendered on the client. This distinction is crucial for optimizing the performance of an application, as it allows developers to decide which parts of the UI should have interactivity and require client-side JavaScript, and which parts can be statically rendered on the server.
By establishing client boundaries, developers can ensure that interactive components are only loaded and executed when necessary. This selective rendering minimizes the amount of code that needs to be sent over the network and parsed by the browser, leading to quicker interactive times and a smoother user experience. Client boundaries also enable better code organization and maintainability by clearly separating concerns between server and client logic.
6. Output of Server Components
The output of server components is a serialized representation of the component's rendered output, which is then sent to the client. This serialization process converts the component into a format that can be transmitted over the network and reconstructed on the client side. The serialized output includes the necessary data and instructions for the client to hydrate the component if interactivity is required.
This approach is highly efficient, as it allows the server to perform complex rendering tasks without involving the client's resources. The client receives a minimal and optimized set of instructions to display the content, which can significantly reduce the time to interactive. Moreover, since server components do not rely on client-side JavaScript, they contribute to a lighter overall application bundle, further enhancing performance.
7. Benefits of React Server Components
// UserList.server.js
import React from 'react';
import db from './database'; // Hypothetical database module
// Server component for fetching and rendering a list of users
function UserList() {
// Directly access the database on the server
const users = db.query('SELECT * FROM users'); // Hypothetical synchronous database query
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
export default UserList;
React Server Components bring a host of benefits to the table, revolutionizing the way developers approach rendering in React applications. One of the most significant advantages is the potential for improved performance, as server components reduce the amount of JavaScript code that needs to be downloaded, parsed, and executed on the client side. This leads to faster page loads and a more responsive user experience, especially on mobile devices and slower networks.
Another benefit is the enhanced data fetching capabilities of server components. Since they run on the server, they can directly access databases and other backend services without the need for additional API calls from the client. This can simplify data fetching logic and reduce latency, resulting in a more efficient data retrieval process. Additionally, server components can help improve code maintainability by allowing developers to write cleaner, more modular code with a clear separation between server and client responsibilities.
8. Combining React Server Components with other React features
React Server Components can be seamlessly integrated with other React features to create powerful and efficient web applications. For instance, they can be used in conjunction with React's state management and context systems to share data between server and client components. This integration allows for a cohesive development experience while still taking advantage of the performance benefits of server rendering.
Furthermore, React Server Components can be combined with existing client-side routing solutions to enable dynamic routing while maintaining the advantages of server rendering. This combination ensures that users receive a fast initial load with the interactivity they expect from a single-page application. By leveraging both server and client capabilities, developers can create applications that are both performant and feature-rich.
9. The Joy of React course
For developers looking to deepen their understanding of React and its ecosystem, including React Server Components, "The Joy of React" course is an excellent resource. This comprehensive course covers the latest React features and best practices, providing learners with the knowledge and skills needed to build modern, high-performance web applications. Through hands-on tutorials and real-world examples, the course explores the intricacies of React, including server components, and how to effectively utilize them in development projects.
10. Future of React Server Components
The future of React Server Components looks promising as the React team continues to innovate and improve the framework. As the community adopts and experiments with server components, we can expect to see new patterns and best practices emerge, further enhancing the capabilities and performance of React applications. The ongoing development of React Server Components is likely to focus on improving the developer experience, optimizing performance, and ensuring seamless integration with the broader React ecosystem.
As web development trends towards more dynamic and complex applications, the role of server components in achieving optimal performance and user experience will become increasingly important. The React community's commitment to embracing these advancements positions React Server Components as a key player in the future of web development, offering developers a powerful tool to build the next generation of web applications.