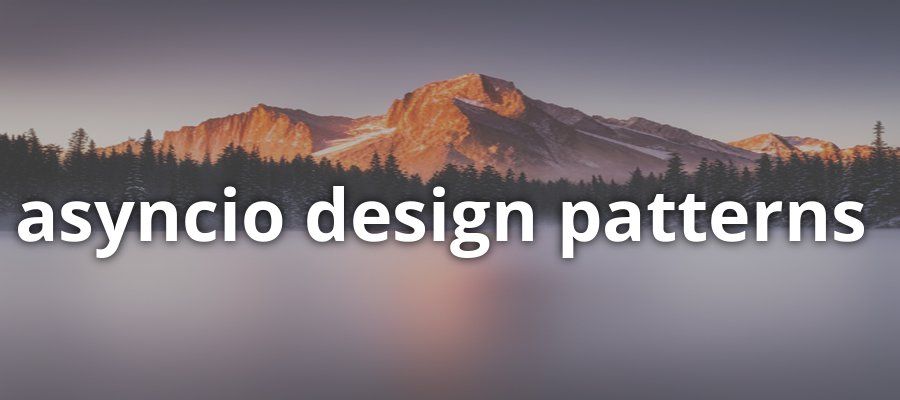
Asyncio Design Patterns
• January 4, 2024
Learn effective asyncio design patterns that harness the power of asyncio to create efficient and robust applications.
Understanding Asyncio: Core Concepts and Patterns
1.1 The Event Loop: Orchestrating Asynchronous Operations
The event loop is the central executor of the asyncio framework, responsible for managing and distributing asynchronous tasks. It operates by continuously collecting and dispatching events to the appropriate coroutine or callback function, effectively enabling concurrent execution without the need for multiple threads or processes. The event loop maintains a queue of tasks, which are instances of coroutines awaiting execution. When a coroutine yields control, typically through an await
expression, the event loop takes over, suspending the coroutine's state and resuming another, thus optimizing the use of the CPU and I/O resources.
In practice, the event loop is initiated using asyncio.run()
or by explicitly declaring an event loop instance with loop = asyncio.get_event_loop()
. The loop then proceeds to execute tasks with loop.run_until_complete()
or loop.run_forever()
, depending on the desired program flow. It is crucial to understand that the event loop is the backbone of asyncio's non-blocking operations, and its efficient management is key to high-performance asynchronous applications.
1.2 Coroutines and Tasks: Building Blocks of Asyncio
Coroutines are the fundamental building blocks of asyncio and represent a powerful abstraction for asynchronous execution. Defined with the async def
syntax, coroutines allow for the suspension and resumption of function execution, making them ideal for I/O-bound and high-level structured network code. When a coroutine awaits on a Future or another coroutine, it effectively signals the event loop to pause its execution and resume later, allowing other operations to proceed in the meantime.
Tasks are a subclass of Future and serve as wrappers around coroutines, facilitating their management and execution in the event loop. They are created by calling asyncio.create_task(coroutine)
, which schedules the coroutine to be run on the event loop and returns a Task object. Tasks can be awaited, yielding control until the task is complete, or they can be used to obtain results or handle exceptions. Understanding the interplay between coroutines and tasks is essential for mastering asyncio's concurrency model.
1.3 Async/Await Syntax: Writing Asynchronous Code
The async
and await
keywords are syntactic elements introduced to write asynchronous code in a more readable and intuitive way. An async
function defines a coroutine, which can include await
expressions to pause its execution until the awaited coroutine or Future completes. This syntax simplifies the chaining of coroutines and the handling of asynchronous operations.
For example, consider the following coroutine:
async def fetch_data():
data = await some_io_task()
return data
Here, fetch_data
is a coroutine that awaits the completion of some_io_task()
, another coroutine or an I/O-bound operation. The await
keyword suspends fetch_data
's execution at this point, allowing the event loop to run other tasks. Once some_io_task()
completes, fetch_data
resumes and returns the result. This pattern is a cornerstone of writing effective and efficient asynchronous code with asyncio.
Effective Asyncio Design Patterns
Asyncio, a Python library for writing concurrent code using the async/await syntax, is an essential tool for modern Python developers. It is particularly useful for I/O-bound and high-level structured network code. This section delves into effective design patterns that harness the power of asyncio to create efficient and robust applications.
2.1 Chaining Coroutines: Sequential Asynchronous Workflows
Chaining coroutines is a fundamental pattern in asyncio that allows developers to execute asynchronous operations in a specific sequence. This pattern is crucial when the output of one coroutine is the input for another, or when operations need to be performed in a particular order.
async def fetch_data():
# Simulate a network operation using sleep
await asyncio.sleep(1)
return {'data': 'sample'}
async def process_data(data):
# Simulate data processing using sleep
await asyncio.sleep(1)
processed_data = data.upper()
return processed_data
async def save_data(processed_data):
# Simulate saving data to a database using sleep
await asyncio.sleep(1)
return f'Data saved: {processed_data}'
async def main():
data = await fetch_data()
processed_data = await process_data(data['data'])
result = await save_data(processed_data)
print(result)
# Run the main coroutine
asyncio.run(main())
In this example, fetch_data
, process_data
, and save_data
are coroutines that must be executed sequentially. The main
coroutine orchestrates the workflow, ensuring that each step is completed before the next begins.
2.2 Using Queues: Managing Tasks and Data Flow
Queues in asyncio provide a way to distribute work across multiple coroutines and manage the flow of data. They are particularly useful in producer-consumer scenarios where you have coroutines producing items that other coroutines consume.
async def producer(queue):
for i in range(5):
# Simulate a producing operation
await asyncio.sleep(1)
await queue.put(f'item {i}')
async def consumer(queue):
while True:
# Wait for an item from the producer
item = await queue.get()
# Process the item
print(f'Processing {item}')
# Notify the queue that the item has been processed
queue.task_done()
async def main():
queue = asyncio.Queue()
# Launch producer and consumer coroutines
producer_task = asyncio.create_task(producer(queue))
consumer_task = asyncio.create_task(consumer(queue))
# Wait until the producer is finished
await producer_task
# Wait until all items have been processed
await queue.join()
# Cancel the consumer task
consumer_task.cancel()
asyncio.run(main())
In the above code, the producer
coroutine puts items into the queue, while the consumer
coroutine takes items out of the queue and processes them. The main
coroutine ensures that the producer and consumer are running and synchronizes their completion.
2.3 Error Handling and Cancellation: Robust Asyncio Applications
Error handling and task cancellation are critical for creating resilient asyncio applications. Properly managing exceptions and cancellations ensures that the application can recover from unexpected states and terminate gracefully.
async def task():
try:
# Simulate a task that may raise an exception
await asyncio.sleep(2)
raise Exception('Task error')
except asyncio.CancelledError:
print('Task was cancelled')
except Exception as e:
print(f'Task raised an exception: {e}')
async def main():
# Create a task and run it
t = asyncio.create_task(task())
await asyncio.sleep(1)
# Cancel the task
t.cancel()
try:
await t
except asyncio.CancelledError:
print('Main also sees the task was cancelled')
asyncio.run(main())
In this example, the task
coroutine is designed to handle both cancellation and exceptions. The main
coroutine starts the task, waits for a moment, and then cancels it. Both coroutines handle the CancelledError
to ensure that the application can respond appropriately to the cancellation request.
By implementing these design patterns, developers can leverage asyncio's full potential to create high-performance, scalable, and maintainable Python applications.
Advanced Asyncio Techniques
3.1 Async Generators and Context Managers: Extending Asyncio's Capabilities
Asyncio's utility in Python's asynchronous programming landscape is further enhanced by the introduction of async generators and context managers. An async generator is a special type of generator that can pause execution before yielding a value, allowing for asynchronous operations within the generator function. This is denoted by the syntax async def
in conjunction with yield
. For example:
async def async_generator():
for i in range(10):
await asyncio.sleep(1)
yield i
Context managers in asyncio are used to manage resources that need to be set up before and cleaned up after asynchronous operations. They are defined using async def
and the async with
statement, ensuring that resources are properly managed even in the face of asynchronous execution. An example of an async context manager is as follows:
class AsyncContextManager:
async def __aenter__(self):
# setup code
return resource
async def __aexit__(self, exc_type, exc, tb):
# cleanup code
pass
Utilizing these constructs allows developers to write more expressive and maintainable asynchronous code, leveraging the full capabilities of asyncio.
3.2 Optimizing Performance: Tuning the Event Loop
The event loop is the core of asyncio's operation, managing the execution of asynchronous tasks and callbacks. Performance tuning of the event loop can lead to significant improvements in the responsiveness and throughput of asyncio-based applications. Developers can adjust various parameters of the event loop, such as the selector policy, which determines how I/O events are monitored and dispatched. Additionally, the event loop's debug mode can be enabled to provide detailed logging and warnings about potential performance issues, such as long-running synchronous functions or excessive callback chaining.
To tune the event loop for optimal performance, one might consider the following adjustments:
# Set a custom selector policy
asyncio.set_event_loop_policy(MySelectorPolicy())
# Enable debug mode for the event loop
loop = asyncio.get_event_loop()
loop.set_debug(True)
By fine-tuning these and other settings, developers can optimize the event loop to better suit the specific needs of their application.
3.3 Integrating with Synchronous Code: Bridging the Async Gap
In many real-world scenarios, asyncio-based applications must interact with legacy synchronous code. Bridging this async-synchronous gap is crucial for maintaining compatibility and ensuring smooth operation. One common approach is to run synchronous functions in a separate thread or process, using asyncio's loop.run_in_executor
method. This allows the synchronous code to execute without blocking the asynchronous event loop.
An example of integrating synchronous code with asyncio is as follows:
def synchronous_function():
# Time-consuming synchronous operation
pass
async def main():
loop = asyncio.get_event_loop()
# Run the synchronous function in a separate thread
await loop.run_in_executor(None, synchronous_function)
This technique ensures that the synchronous code's execution does not impede the progress of the asynchronous event loop, allowing both to coexist within the same application.
Asyncio in Practice: Real-World Applications
4.1 Asynchronous Networking: Building Scalable IO-bound Applications
In the realm of asynchronous networking, asyncio
provides a robust foundation for building scalable IO-bound applications. By leveraging the non-blocking nature of asyncio
, developers can create networked applications capable of handling a multitude of simultaneous connections without the overhead of multi-threading or multi-processing. This is particularly advantageous in scenarios where the application must maintain many open connections to various services, each with potential latency in response times.
The cornerstone of asyncio
networking is the event loop, which monitors and dispatches events to the appropriate coroutine when the operation can proceed. For instance, when a network request is made, the coroutine yields control back to the event loop, allowing other tasks to run while waiting for the network response. This pattern maximizes the utilization of CPU resources and minimizes idle time.
To illustrate, consider a server implementation that handles incoming client connections. Each connection can be managed by a coroutine, and with asyncio
's create_server
utility, the server can service hundreds or even thousands of clients concurrently. Here's a simplified example:
import asyncio
async def handle_client(reader, writer):
request = await reader.read(100)
# Process the request...
response = b'HTTP/1.1 200 OK\r\n\r\nHello, World!'
writer.write(response)
await writer.drain()
writer.close()
async def main():
server = await asyncio.start_server(handle_client, '127.0.0.1', 8080)
await server.serve_forever()
asyncio.run(main())
In this example, handle_client
is a coroutine that processes client requests. The start_server
function initiates the server and listens for incoming connections, which are then handled concurrently by the event loop.
4.2 Asyncio with Databases: Non-blocking Database Operations
Database operations are often a bottleneck in application performance, particularly when dealing with IO-bound tasks such as querying or writing to a database. asyncio
can be used to perform non-blocking database operations, thereby improving the overall efficiency of the application. Libraries such as aiomysql
and aiopg
provide asynchronous interfaces to MySQL and PostgreSQL databases, respectively.
When using asyncio
with databases, it is crucial to use an asynchronous database driver compatible with the asyncio
event loop. This ensures that database operations do not block the event loop and that other coroutines can continue executing while waiting for the database operation to complete.
Here's an example of performing an asynchronous query using aiopg
:
import asyncio
import aiopg
dsn = 'dbname=example user=postgres password=secret'
async def go():
async with aiopg.create_pool(dsn) as pool:
async with pool.acquire() as conn:
async with conn.cursor() as cur:
await cur.execute("SELECT * FROM my_table")
ret = []
async for row in cur:
ret.append(row)
return ret
loop = asyncio.get_event_loop()
loop.run_until_complete(go())
In this snippet, the go
coroutine establishes a connection pool, acquires a connection, and performs a query. The use of async with
ensures that resources are released back to the pool once the operation is complete.
4.3 Testing Asyncio Code: Strategies and Best Practices
Testing asyncio
code requires a different approach compared to synchronous code. The asynchronous nature of the code under test means that the testing framework must be aware of the event loop and able to run coroutines. The pytest-asyncio
plugin for pytest
is one such tool that facilitates the testing of asyncio
coroutines.
A best practice for testing asyncio
code is to ensure that each test case is independent and that the event loop is in a clean state before each test. This can be achieved by using fixtures that set up and tear down the event loop for each test case.
An example of an asyncio
test case using pytest
and pytest-asyncio
might look like this:
import pytest
import asyncio
@pytest.mark.asyncio
async def test_some_async_function():
result = await some_async_function()
assert result == expected_result
The @pytest.mark.asyncio
decorator tells pytest
to run the test as an asynchronous coroutine. This allows for the use of await
within the test to call asynchronous functions and wait for their completion.
In conclusion, asyncio
is a powerful tool for building scalable and efficient IO-bound applications, integrating with databases in a non-blocking manner, and writing testable asynchronous code. By understanding and applying the design patterns and best practices of asyncio
, developers can leverage the full potential of asynchronous programming in Python.
Choosing the Right Asyncio Strategy
5.1 When to Use Asyncio: Use Cases and Considerations
Asyncio is an asynchronous programming framework that excels in situations where I/O-bound operations are prevalent. It is particularly effective when a program must maintain high responsiveness under the load of concurrent I/O-bound tasks, such as handling numerous web requests or managing multiple network connections simultaneously. The use of asyncio should be considered when the performance bottleneck of an application is not CPU computations but rather waiting for I/O operations to complete.
The decision to implement asyncio must also take into account the complexity it introduces into the codebase. While asyncio can lead to significant performance improvements, it requires a solid understanding of its event loop, coroutines, and the async/await syntax. Developers must weigh the benefits of increased I/O efficiency against the potential for more complex code and the necessity for asynchronous-aware libraries.
5.2 Comparing Asyncio with Other Concurrency Models
Asyncio is not the only concurrency model available to Python developers. Other models, such as threading and multiprocessing, serve different purposes and have distinct advantages and disadvantages. Threading allows for concurrent execution of code segments and is suitable for I/O-bound tasks, but it is limited by the Global Interpreter Lock (GIL) in CPython, which prevents multiple native threads from executing Python bytecodes simultaneously. Multiprocessing circumvents the GIL by running tasks in separate processes, thus enabling parallel CPU computation.
When comparing asyncio with these models, it is crucial to understand that asyncio provides a single-threaded, single-process design that uses non-blocking I/O calls, allowing the event loop to switch tasks efficiently without the overhead of context switching found in threading or the inter-process communication required by multiprocessing. This makes asyncio particularly well-suited for high I/O scenarios where tasks can be performed concurrently without the need for parallel execution.
5.3 Future of Asyncio: Trends and Developments
The asyncio module has rapidly evolved since its introduction, and its future looks promising. The Python community continues to embrace asynchronous programming, leading to the development of more libraries and frameworks that are compatible with asyncio's paradigms. The ongoing enhancements to the asyncio module itself and the broader Python language are expected to further simplify asynchronous programming, making it more accessible and performant.
One trend is the increasing integration of asyncio into web frameworks, allowing for the development of scalable web applications. Additionally, the async/await syntax has become more prevalent, and its usage is expanding beyond the scope of I/O-bound tasks. As Python's asynchronous ecosystem matures, we can anticipate more robust tooling and improved interoperability with synchronous code, which will solidify asyncio's position as a key component in Python's concurrency toolkit.