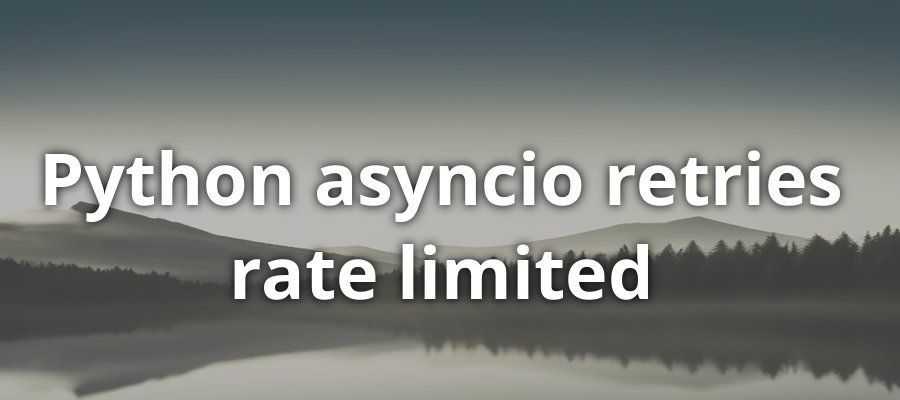
Python asyncio retries rate limited
• March 4, 2024
A guide for learning how about rate limiting for Python asyncio retries and how to implement an async python client rate limiter.
Understanding Python Asyncio for Rate Limiting
1.1 Introduction to Asyncio and Rate Limiting
Python's asyncio library is a cornerstone of asynchronous programming, enabling the execution of multiple I/O-bound tasks without the need for multi-threading. This is particularly useful in scenarios where applications need to make numerous external API calls or handle a large volume of network requests concurrently. Rate limiting, on the other hand, is a crucial mechanism to control the rate of requests sent or received by a program. It is often implemented to comply with API usage policies, prevent abuse, and manage resource utilization effectively.
When combined, asyncio and rate limiting allow developers to build efficient and respectful applications that maximize throughput without overstepping boundaries set by external services or overwhelming resources. This section delves into the integration of asyncio with rate limiting strategies, providing a foundation for understanding how to implement these concepts in Python applications.
1.2 Key Concepts: Async/Await, Throttling, and Retries
Async/Await
The async/await syntax introduced in Python 3.5 simplifies the writing and maintenance of asynchronous code. An async
function is a coroutine that can be paused and resumed at await points, allowing other tasks to run in the meantime. This non-blocking behavior is essential for asynchronous programming, enabling the efficient management of I/O operations.
async def fetch_data(session, url):
async with session.get(url) as response:
return await response.text()
Throttling
Throttling is a form of rate limiting where the execution of requests is evenly spread over time to avoid bursts of traffic. This is particularly useful when interacting with APIs that impose limits on the number of requests per second or minute. Implementing throttling requires a mechanism to monitor and control the rate of requests made by the application.
Retries
Retries are a strategy to handle requests that fail due to temporary issues, such as network timeouts or rate limit errors. An effective retry mechanism involves waiting for a certain period before attempting the request again, possibly with exponential backoff to reduce the load on the server and increase the likelihood of success on subsequent attempts.
Implementing retries in an asyncio environment requires careful consideration to avoid blocking the event loop. The use of asynchronous sleep (await asyncio.sleep(delay)
) allows the program to pause execution of a coroutine and resume it later, providing an opportunity to implement retries without impacting the responsiveness of the application.
Together, these concepts form the basis of efficient and responsible asynchronous programming, enabling developers to build applications that are both performant and compliant with external constraints.
Implementing Rate Limiting with Asyncio
Using httpx and aiometer for Async Requests
In the realm of Python programming, particularly when dealing with asynchronous operations, the httpx
and aiometer
libraries emerge as pivotal tools for executing HTTP requests concurrently. This subsection delves into the utilization of these libraries to implement rate limiting, a crucial aspect in preventing the overloading of servers and adhering to API usage policies.
httpx
, a fully featured HTTP client for Python 3, supports asynchronous requests out of the box. Coupled with aiometer
, a library designed to manage asynchronous concurrency and rate limiting, developers can efficiently control the rate at which HTTP requests are made. The following code snippet demonstrates a basic setup for making asynchronous requests with rate limiting:
import httpx
import asyncio
from aiometer import amap
async def fetch(url, client):
response = await client.get(url)
return response
async def main(urls):
async with httpx.AsyncClient() as client:
# Define the rate limit (e.g., 10 requests per second)
async with aiometer.amap(fetch, urls, client=client, max_at_once=10, max_per_second=10) as responses:
return [response async for response in responses]
urls = ["http://example.com"] * 100 # List of URLs to fetch
results = asyncio.run(main(urls))
In this example, aiometer.amap
is used to asynchronously map the fetch
function over a list of URLs, with the httpx.AsyncClient
passed as an argument to handle HTTP requests. The parameters max_at_once
and max_per_second
are crucial for rate limiting, ensuring that no more than 10 requests are made concurrently and no more than 10 requests are made per second, respectively.
Handling Rate-Limited Requests in Python
When implementing rate limiting in Python, especially in asynchronous environments, handling 429 Too Many Requests errors becomes an essential consideration. These errors indicate that the rate limit has been exceeded. Proper handling involves retrying the request after a delay, a process that can be automated using httpx
and aiometer
.
Consider the scenario where an API endpoint imposes a rate limit, and exceeding this limit results in a 429 response. The following approach demonstrates how to handle such responses by retrying the request after a specified delay:
import httpx
import asyncio
from aiometer import amap
async def fetch_with_retry(url, client, retries=3, backoff_factor=0.5):
for attempt in range(retries):
response = await client.get(url)
if response.status_code != 429:
return response
# Calculate delay using a simple exponential backoff strategy
delay = backoff_factor * (2 ** attempt)
await asyncio.sleep(delay)
raise Exception("Rate limit exceeded, retries failed.")
async def main(urls):
async with httpx.AsyncClient() as client:
async with aiometer.amap(fetch_with_retry, urls, client=client, max_at_once=10, max_per_second=10) as responses:
return [response async for response in responses]
urls = ["http://example.com"] * 100
results = asyncio.run(main(urls))
In this enhanced example, fetch_with_retry
attempts to make a request up to a specified number of retries (retries=3
) whenever a 429 status code is encountered. The delay between retries increases exponentially (backoff_factor=0.5
), a strategy known as exponential backoff, which helps to mitigate further rate limit violations.
By integrating httpx
and aiometer
with appropriate error handling and retry strategies, developers can effectively manage rate-limited requests in Python, ensuring compliance with API rate limits while minimizing the impact on application performance.
Advanced Techniques and Best Practices
Exploring Back-off Strategies for Efficient Retries
When dealing with rate-limited APIs in Python, implementing efficient retry mechanisms is crucial. A sophisticated approach involves the use of back-off strategies, which dynamically adjust the wait time between retries to optimize both server load and the likelihood of a successful request without hitting the rate limit again. The core principle behind back-off strategies is to reduce the rate of requests in response to server feedback indicating overload, typically manifested as HTTP 429 (Too Many Requests) errors.
The simplest form of back-off is the linear back-off, where the wait time increases by a fixed amount after each retry. However, more complex and effective strategies, such as exponential back-off, are widely recommended. In exponential back-off, the wait time doubles after each unsuccessful attempt, optionally with a random jitter added to prevent synchronized retries from multiple clients. This method is particularly effective in distributed systems where numerous clients are interacting with the same server.
Implementing exponential back-off in Python can be achieved with the asyncio
library, leveraging the asyncio.sleep()
function to introduce delay. A basic exponential back-off algorithm would look something like this:
import asyncio
import random
async def request_with_backoff(request, max_attempts=5):
for attempt in range(max_attempts):
try:
# Replace with actual request logic
response = await request()
return response
except RateLimitException as e:
wait = 2 ** attempt + random.random() # Exponential back-off plus jitter
await asyncio.sleep(wait)
raise MaxRetriesExceededError
This snippet demonstrates a function that attempts a request with exponential back-off, incorporating jitter to minimize collision. It's a foundational technique for efficiently managing retries in an asynchronous environment.
Utilizing Python Libraries for Enhanced Rate Limiting
Beyond custom implementations, several Python libraries offer built-in support for rate limiting and retries with advanced back-off strategies. Libraries such as httpx
and aiometer
provide asynchronous request capabilities, while also allowing for sophisticated control over rate limiting and retry logic.
httpx
, a powerful HTTP client for Python, supports asynchronous requests and can be combined with aiometer
to manage rate limiting. aiometer
offers a straightforward way to apply rate limits over asynchronous operations, using a token bucket algorithm to control the rate of operations. Here's how you might use these libraries together to perform rate-limited requests:
import httpx
import aiometer
import asyncio
async def fetch_url(client, url):
response = await client.get(url)
return response
async def main(urls):
async with httpx.AsyncClient() as client:
await aiometer.run_on_each(fetch_url, urls, client=client, max_at_once=10, max_per_second=10)
urls = ["http://example.com" for _ in range(100)]
asyncio.run(main(urls))
In this example, aiometer.run_on_each
is used to fetch multiple URLs concurrently, adhering to specified rate limits. This approach simplifies the implementation of efficient, scalable rate limiting for HTTP requests in Python applications.
By leveraging these advanced techniques and best practices, developers can effectively manage rate limits, ensuring robust and resilient interaction with rate-limited APIs.
Case Studies and Real-World Applications
Analyzing Successful Implementations
In the realm of software development, particularly in web services and APIs, managing the flow of requests is crucial to maintain system integrity, performance, and user experience. Python's asyncio library, combined with strategic rate limiting, has proven to be an effective tool in achieving these goals. This section delves into real-world applications where asyncio and rate limiting have been successfully implemented, showcasing the versatility and efficiency of these approaches.
One notable example involves a large-scale web application that serves millions of users daily. The developers faced challenges with their API being overwhelmed during peak usage times, leading to degraded performance and timeouts. By integrating asyncio into their Python backend and applying rate limiting to their API endpoints, they were able to significantly reduce the load on their servers. This was achieved by asynchronously handling incoming requests and throttling them based on predefined limits, ensuring that the system remained responsive and stable even under heavy load.
Another case study comes from a financial technology company that processes high volumes of data requests from various sources. The nature of their business requires real-time data processing and delivery, making system efficiency paramount. The implementation of asyncio for asynchronous data processing, coupled with a dynamic rate limiting algorithm, allowed them to optimize their data throughput. This not only improved their system's performance but also enhanced the accuracy and timeliness of the data provided to their clients.
These examples underscore the effectiveness of Python's asyncio library and rate limiting in managing high-concurrency environments. By allowing for non-blocking asynchronous operations and controlling the rate of requests, developers can ensure their applications remain scalable, resilient, and efficient.
Overcoming Challenges in Rate Limiting Scenarios
Despite the clear benefits, implementing rate limiting with asyncio presents its own set of challenges. This subsection explores common obstacles encountered in such scenarios and how they were addressed, providing insights into best practices and lessons learned.
One challenge is determining the optimal rate limits for different endpoints or services. Setting these limits too low can unnecessarily restrict legitimate usage, while too high a limit may fail to protect the system from overload. A social media analytics platform faced this dilemma and resolved it by employing adaptive rate limiting. This approach involves dynamically adjusting rate limits based on current system load and historical usage patterns, using asyncio to manage the asynchronous evaluation and enforcement of these limits. This strategy enabled them to balance system protection with user access needs effectively.
Another issue is handling rate-limited requests in a way that minimizes impact on the user experience. A cloud storage service tackled this by implementing a retry mechanism with exponential backoff, using asyncio to manage the scheduling of retries. When a request is rate-limited, it is automatically retried after a delay, with the delay period increasing exponentially on subsequent retries. This method reduces the likelihood of overwhelming the server with repeated requests while increasing the chances of the request eventually being processed.
These case studies illustrate the complexities of implementing rate limiting in asynchronous systems and the innovative solutions developers have devised to overcome them. By sharing these experiences, the aim is to provide valuable insights and guidance for others facing similar challenges in their projects.
Conclusion
Summary of Key Takeaways
The exploration of Python's asyncio library for implementing rate limiting has underscored its significance in managing the flow of requests to web services. By leveraging asyncio, developers can efficiently handle I/O-bound and high-level structured network code. The introduction to asyncio and rate limiting set the foundation, emphasizing the importance of asynchronous programming in Python for optimizing web requests and server responses. Key concepts such as async/await, throttling, and retries were delineated, providing a clear understanding of the mechanisms behind asyncio's operation.
Implementing rate limiting with asyncio was further elaborated through practical examples, utilizing libraries like httpx and aiometer. This section demonstrated how to make asynchronous requests and handle rate-limited responses, showcasing the practical application of the concepts discussed. Advanced techniques and best practices, including back-off strategies and the utilization of Python libraries for enhanced rate limiting, were also covered. These strategies are crucial for developing resilient applications that can efficiently manage request rates and maintain service availability.