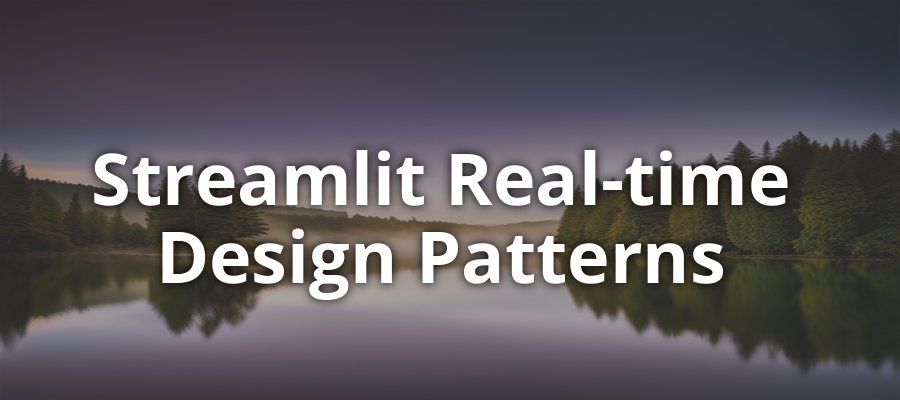
Streamlit Real-time Design Patterns: Creating Interactive and Dynamic Data Visualizations
• January 6, 2024
Learn how to implement real-time design patterns in Streamlit for creating interactive and dynamic data visualizations. Explore techniques for animating line charts, integrating WebRTC for real-time video processing.
Understanding Streamlit for Real-time Applications
Streamlit is an open-source app framework that is a go-to for many developers when it comes to creating data applications with ease. Its design philosophy emphasizes simplicity, ease of use, and the ability to create beautiful web applications with minimal effort. However, when it comes to real-time applications, there are specific considerations and patterns that developers must understand to effectively leverage Streamlit's capabilities. This section delves into the core concepts of Streamlit, its execution model, and how it handles real-time data, providing a foundation for building responsive and dynamic applications.
1.1 Core Concepts of Streamlit
Streamlit operates on the principle of scripts that run from top to bottom, where each script execution reflects a "run" of the application. The framework is built around the concept of widgets, which are interactive elements that capture user input and are used to display information or control the flow of the application. Streamlit's caching mechanisms are pivotal for performance, allowing expensive computations to be memoized and reused across runs. The simplicity of Streamlit lies in its declarative syntax, where the layout and interactivity of the application are defined through straightforward Python code, abstracting away the complexities of web development.
1.2 Streamlit's Execution Model
The execution model of Streamlit is unique in that it re-runs the entire script whenever a user interaction occurs. This means that any change in the widget state, triggered by user input, will cause the script to execute from the top, updating the app with the new state. This model simplifies state management but also poses challenges for real-time applications where continuous data streams are involved. To address this, Streamlit provides mechanisms such as st.empty()
for placeholder elements and st.cache()
to persist data across runs, ensuring that real-time updates do not lead to redundant computations or flickering UI elements.
placeholder = st.empty()
# Inside a loop or a function that updates in real-time
placeholder.chart(updated_data)
1.3 Real-time Data Handling in Streamlit
Handling real-time data in Streamlit requires a nuanced approach to ensure that the UI updates smoothly and efficiently. Streamlit's native support for WebSockets facilitates real-time bi-directional communication between the client and server. Developers can use Streamlit's add_rows
method to append data to existing elements without re-running the entire script, which is crucial for displaying live data feeds. Additionally, Streamlit's integration with external libraries like streamlit-webrtc
allows for handling real-time media streams, expanding the framework's capabilities to include video and audio processing.
# Example of real-time data update
dataframe = st.empty()
for data in real_time_data_stream():
dataframe.add_rows(data)
In summary, understanding Streamlit's core concepts, execution model, and real-time data handling strategies is essential for developers aiming to build interactive and responsive real-time applications. With this knowledge, developers can harness the full potential of Streamlit to create powerful data-driven applications with live updates and real-time interactivity.
Design Patterns for Streamlit Real-time Dashboards
Streamlit has emerged as a powerful tool for building interactive and real-time dashboards with Python. Its simplicity and ability to integrate with the Python data stack make it an ideal choice for developers looking to create live data dashboards without extensive front-end development experience. This section delves into the design patterns that can be leveraged to build efficient and user-friendly real-time dashboards using Streamlit.
2.1 Building Live Data Dashboards
To construct a live data dashboard in Streamlit, one must first understand the core components that constitute its architecture. The primary element is the data source, which can range from APIs delivering real-time streams to databases that are updated continuously. Streamlit facilitates the integration of these data sources through its caching mechanisms and native support for Python's data manipulation libraries.
import streamlit as st
import pandas as pd
from streamlit_autorefresh import st_autorefresh
# Function to fetch live data
@st.experimental_memo
def get_live_data():
# Code to fetch and return live data
pass
# Auto-refresh the dashboard to update the data
st_autorefresh(interval=5000, key='data_refresh')
# Display live data
live_data = get_live_data()
st.write(live_data)
In the snippet above, st.experimental_memo
is used to cache the data and minimize unnecessary data fetching, while st_autorefresh
from the streamlit_autorefresh
package is employed to refresh the dashboard at specified intervals, ensuring the data displayed is up-to-date.
2.2 Optimizing Performance for High-frequency Updates
Performance optimization is critical when dealing with high-frequency data updates. Streamlit provides several strategies to enhance performance, such as minimizing computational load, using efficient data structures, and optimizing data transfer between the server and the client.
One effective approach is to use delta updates, which only send the changes in data rather than the entire dataset. This can be achieved by strategically structuring the data flow and employing Streamlit's native methods for updating specific components.
# Assuming 'live_data' is a pandas DataFrame with real-time stock prices
price_chart = st.empty()
# Update chart with new data using delta updates
price_chart.line_chart(live_data['price'])
In the example, st.empty()
is used to create a placeholder for the chart, which can be updated with new data without re-rendering the entire dashboard, thus improving performance.
2.3 User Interface Considerations
The user interface (UI) of a real-time dashboard should be intuitive and responsive. Streamlit's widgets and layout options enable developers to create a seamless user experience. Key considerations include the arrangement of visual components, the use of space, and the provision of interactive elements that allow users to filter and manipulate the data presented.
# Sidebar for user inputs
with st.sidebar:
selected_metric = st.selectbox('Select Metric', ['Temperature', 'Humidity', 'Pressure'])
# Main dashboard layout
col1, col2 = st.columns(2)
with col1:
st.metric(label="Current Value", value=live_data[selected_metric].iloc[-1])
with col2:
st.line_chart(live_data[selected_metric])
The code above demonstrates the use of a sidebar for user input and the organization of the dashboard into columns, which enhances the UI by allowing users to select metrics and view corresponding data visualizations.
By adhering to these design patterns, developers can build robust real-time dashboards with Streamlit that not only perform well under the demands of live data but also provide an engaging and informative experience for users.
Advanced Streamlit Features for Real-time Interactivity
Streamlit has emerged as a powerful tool for building interactive and real-time applications with ease. This section delves into advanced features that enhance Streamlit's capabilities for real-time interactivity, focusing on event handling, WebRTC integration, and leveraging asyncio for concurrent operations.
3.1 Event Handling and Callbacks
Streamlit's architecture is designed to rerun scripts from top to bottom with each user interaction. However, real-time applications often require more granular control over event handling and callbacks. Streamlit addresses this by allowing developers to define events and associate them with specific callback functions. This is achieved using Streamlit's st.button
and st.form_submit_button
for triggering events, and decorators such as st.cache
to manage state and optimize performance.
For instance, a button click in Streamlit can be handled as follows:
if st.button('Click me'):
handle_event()
Where handle_event
is a function defined to execute a particular logic. This pattern is essential for real-time applications where user interactions must trigger immediate and specific responses within the application.
3.2 Integrating WebRTC for Video Processing
WebRTC (Web Real-Time Communication) is a pivotal technology for enabling real-time communication capabilities in web applications. Streamlit's integration with WebRTC opens up possibilities for real-time video processing, which is critical for applications in fields such as computer vision and telemedicine.
To integrate WebRTC into a Streamlit application, developers can use the streamlit-webrtc
component, which facilitates the exchange of video and audio streams between clients and servers. The component abstracts the complexity of WebRTC's signaling process, offering a simplified API for developers. Here's a basic example of how to use the streamlit-webrtc
component:
import streamlit as st
from streamlit_webrtc import webrtc_streamer
webrtc_streamer(key="example")
This code snippet initializes a WebRTC session within the Streamlit app, allowing users to send and receive video streams. Advanced use cases might involve processing the video stream with machine learning models to perform tasks such as object detection or facial recognition in real-time.
3.3 Leveraging Asyncio for Concurrent Operations
Asyncio is a Python library that provides a framework for writing concurrent code using the async/await syntax. Streamlit applications can leverage asyncio to handle I/O-bound and high-level structured network code. This is particularly useful for real-time applications that require non-blocking operations or have to manage multiple asynchronous tasks concurrently.
For example, Streamlit can use asyncio to fetch data from APIs or databases without blocking the main thread, ensuring the application remains responsive. Here's a simple demonstration of using asyncio within Streamlit:
import asyncio
import streamlit as st
async def get_data():
# Asynchronous code to fetch data
await asyncio.sleep(1) # Simulate I/O operation
return "Data fetched"
data = asyncio.run(get_data())
st.write(data)
In this example, get_data
is an asynchronous function that simulates a data-fetching operation. The asyncio.run
function is used to run the coroutine, and the fetched data is displayed in the Streamlit app.
By incorporating these advanced features, Streamlit developers can create sophisticated real-time applications that are interactive, responsive, and capable of handling complex tasks efficiently.
4. Troubleshooting and Optimizing Streamlit Apps
4.1 Common Issues and Solutions
Streamlit's ease of use and rapid development capabilities are accompanied by a unique set of challenges. A common issue encountered by developers is the set_page_config
error, which arises when the command is not the first Streamlit function called in the script. The correct usage is as follows:
st.set_page_config(page_title='My App', layout='wide')
df = get_data()
Another frequent problem is related to the use of loops, particularly when integrating input components. Streamlit's execution model does not support adding input widgets from within an asynchronous thread. To circumvent this, developers can utilize st.empty
to create placeholders for live updating charts, ensuring that input widgets are rendered correctly.
For instance, to maintain a responsive user interface with a select box under a live chart:
placeholder = st.empty()
# ... Data processing and chart updates
placeholder.selectbox('Choose a value', options)
4.2 Performance Tuning and Best Practices
Optimizing Streamlit applications for performance involves several best practices. One key strategy is to minimize the computational load by caching expensive functions using the @st.cache
decorator. This prevents the re-execution of functions with unchanged parameters, thus saving valuable processing time and resources.
@st.cache
def get_expensive_data(parameters):
# Time-consuming data retrieval and processing
return data
Another aspect of performance tuning is the judicious use of data operations. Streamlit's API is evolving to support a variety of data manipulations. For example, the proposed st.Data
object would allow direct manipulation of dataframes or arrays, enabling operations like appending, removing, or replacing rows without the need for specific element methods like st.add_rows
.
data = st.Data(my_dataframe)
st.line_chart(data)
# Append rows
data += more_rows
# Remove rows from the head
del data[:10]
# Replace a specific row
data[50] = new_row
Implementing these performance optimizations can significantly enhance the responsiveness and efficiency of Streamlit applications, particularly in real-time scenarios.
5. Streamlit in Practice: Case Studies and Examples
Streamlit, an open-source app framework, is a compelling tool for building and sharing data applications. It has been widely adopted for its ease of use and flexibility, allowing data scientists and engineers to turn data scripts into shareable web apps quickly. This section delves into practical implementations of Streamlit, focusing on real-time applications and interactive data visualizations.
5.1 Real-time SCADA Systems with Streamlit
Supervisory Control and Data Acquisition (SCADA) systems are crucial for industrial automation, providing a high-level interface to control processes and machinery. Streamlit's ability to handle real-time data makes it an excellent choice for developing SCADA systems. By leveraging Streamlit's reactive programming model, developers can create dashboards that update in real-time as new data streams in from sensors and actuators.
For instance, consider a Streamlit application designed to monitor a manufacturing line. The app could display real-time metrics such as temperature, pressure, and throughput from various sensors. Using Streamlit's st.line_chart
or st.area_chart
, the data can be visualized as it updates, giving operators immediate feedback on the system's status.
import streamlit as st
import pandas as pd
import numpy as np
# Simulate real-time sensor data
data = pd.DataFrame(
np.random.randn(50, 3),
columns=['Temperature', 'Pressure', 'Throughput']
)
st.line_chart(data)
This snippet demonstrates how a Streamlit app could render a line chart that updates as new data is received. The simplicity of integrating real-time data streams with interactive visualizations is a testament to Streamlit's capabilities in industrial applications.
5.2 Interactive Data Visualization for Live Feeds
Data visualization is a powerful tool for understanding complex data. Streamlit enhances this by allowing for interactive visualizations that can update with live data feeds. This is particularly useful in scenarios such as financial markets, social media analytics, or IoT device monitoring, where data is continuously generated.
A case study in this context could be a financial dashboard that tracks market indices in real-time. Streamlit can be used to create an interactive chart that updates as new ticker information is received from a market data API. The st.altair_chart
function, for example, can be used to create sophisticated and interactive charts.
import streamlit as st
import altair as alt
from vega_datasets import data
# Load sample data
source = data.stocks()
# Create an interactive chart
chart = (
alt.Chart(source)
.mark_line()
.encode(x='date:T', y='price:Q', color='symbol:N')
.interactive()
)
st.altair_chart(chart, use_container_width=True)
In this example, the Streamlit app uses Altair to render an interactive line chart of stock prices over time. Users can zoom in and out, pan across the chart, and explore the data in a way that static charts do not permit.
Streamlit's ability to integrate with various charting libraries and handle real-time data updates makes it an invaluable tool for creating dynamic and responsive data visualizations. The case studies presented in this section illustrate the practical applications of Streamlit in real-world scenarios, showcasing its potential to revolutionize the way we interact with data.