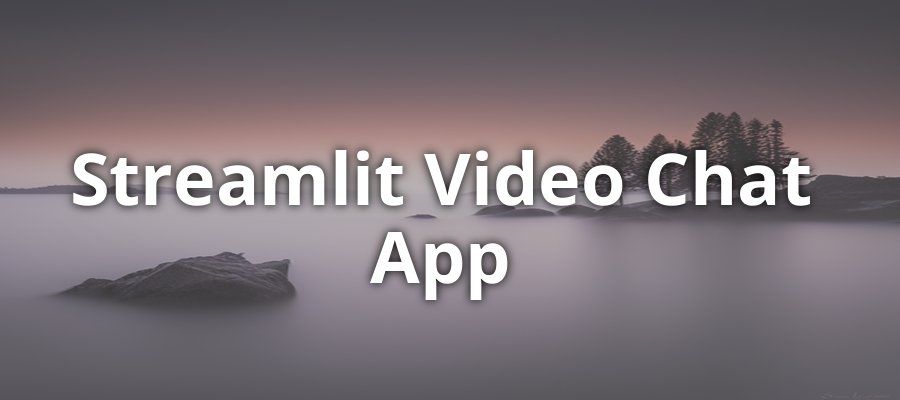
Streamlit Video Chat App with Realtime Filters
• January 6, 2024
Learn how to build a Streamlit video chat app with realtime snapchat-like filters. This article covers the steps to create a seamless video chat experience.
Introduction to Streamlit Video Chat App
Streamlit has emerged as a powerful tool for rapid development of data applications with its easy-to-use syntax and wide array of widgets. Among its many capabilities, the integration of real-time video chat functionality using the streamlit-webrtc
component has opened new avenues for developers to create interactive and engaging applications. This section delves into the Streamlit Video Chat App, exploring its core features and the underlying Streamlit framework that enables such innovative capabilities.
Exploring the Core Features
The Streamlit Video Chat App leverages the streamlit-webrtc
component to facilitate real-time video communication. This component is built on top of WebRTC, a technology that supports browser-to-browser communication, including video and audio streaming, without the need for additional plugins. The core features of the Streamlit Video Chat App include:
- Real-time video and audio streaming: Users can engage in live video chats with low latency, which is crucial for maintaining a seamless conversation flow.
- Media stream manipulation: Developers can apply real-time video and audio processing, such as face detection or applying filters, using libraries like OpenCV.
- Session state management: The
streamlit-server-state
library allows for the preservation of state across different user sessions, enabling features like chat history or user-specific data retention.
The combination of these features within the Streamlit ecosystem allows for the creation of robust video chat applications that can be customized and extended to meet various use cases.
Understanding the Streamlit Framework
Streamlit's framework is designed to simplify the process of building and deploying data applications. It provides a set of high-level abstractions for designing user interfaces, handling user input, and displaying data. Key aspects of the Streamlit framework that are particularly relevant to developing a video chat application include:
- Widgets: Streamlit offers a variety of widgets, such as buttons, sliders, and text inputs, which can be used to create interactive user interfaces with minimal code.
- Components: The framework supports custom components, allowing developers to integrate third-party libraries and create new functionalities, such as the
streamlit-webrtc
component used for video chat. - Deployment: Streamlit apps can be easily deployed on various platforms, including Streamlit Sharing and Streamlit for Teams, facilitating quick sharing and collaboration.
Understanding the Streamlit framework is essential for developers looking to build a video chat application, as it provides the necessary tools and components to create a fully functional and user-friendly app.
Setting Up Your Streamlit Video Chat Environment
In this section, we will guide you through the initial setup required to create a Streamlit Video Chat App. This setup is a critical foundation for developing a robust and functional video chat application using the Streamlit framework.
2.1 Installation and Required Packages
To begin, you must ensure that your development environment is equipped with Python 3.6 or later. Streamlit's video chat capabilities are extended by the streamlit-webrtc
package, which leverages WebRTC technology to facilitate real-time communication. Additionally, the streamlit-server-state
package is utilized to manage state across different user sessions on the server.
The installation process commences with the creation of a virtual environment, which is recommended to avoid conflicts with existing Python packages. Execute the following commands in your terminal:
python3 -m venv streamlit-venv
source streamlit-venv/bin/activate
With the virtual environment activated, install Streamlit and the necessary packages using pip:
pip install streamlit streamlit-webrtc streamlit-server-state
Ensure that all packages are installed without errors before proceeding to the next step.
2.2 Running the Video Chat App Locally
Once the required packages are installed, you can run the Streamlit Video Chat App locally for development and testing purposes. To initiate the app, navigate to the directory containing your Streamlit application script and run:
streamlit run your_app.py
Replace your_app.py
with the actual filename of your Streamlit application. Upon execution, Streamlit will start a local server, and you should see output in the terminal indicating the local URL, typically http://localhost:8501
, where the app is being served.
Before inviting users to connect, it is imperative to verify that the application is functioning correctly in the local environment. Test the video and audio transmission, the interface responsiveness, and the real-time communication features thoroughly.
By following these steps, you have successfully set up your local Streamlit Video Chat Environment. This environment serves as a sandbox for further development, allowing you to integrate additional features and refine the app's functionality.
Developing with Streamlit-webrtc: Real-time Video Processing
Streamlit has emerged as a powerful tool for rapid development of data applications, but its native capabilities for real-time video processing have been limited. The integration of WebRTC with Streamlit, through the streamlit-webrtc component, has opened new avenues for developers to build interactive applications that require real-time video streaming and processing capabilities.
3.1 Integrating WebRTC in Streamlit
The integration of WebRTC into Streamlit is facilitated by the streamlit-webrtc component, which acts as a bridge between the Streamlit frontend and the WebRTC protocol. This component leverages the aiortc library, a Python implementation of WebRTC, to establish peer-to-peer connections capable of streaming video and audio data. To incorporate the streamlit-webrtc component into a Streamlit application, developers must first install the necessary dependencies, including aiortc and streamlit-webrtc itself.
pip install streamlit-webrtc aiortc
Once the installation is complete, developers can import the webrtc_streamer
function from the streamlit-webrtc package and use it within their Streamlit application to create a video streamer component.
from streamlit_webrtc import webrtc_streamer
webrtc_streamer(key="example")
This simple integration process allows developers to quickly set up a video chat or streaming service within their Streamlit applications. The component handles the complex aspects of WebRTC, such as signaling and peer connection management, abstracting these details away from the developer.
3.2 Handling Media Streams and Video Processing
The real-time processing of media streams is a critical aspect of any video chat application. Streamlit-webrtc provides a framework for handling incoming video frames and applying custom processing functions, such as computer vision algorithms or machine learning models.
Developers can define a class that extends the VideoTransformerBase
class provided by streamlit-webrtc. This class should implement a transform
method, which receives a frame as input and returns the processed frame.
from streamlit_webrtc import VideoTransformerBase, webrtc_streamer
class VideoFilter(VideoTransformerBase):
def transform(self, frame):
# Apply video processing here
# For example, convert the image to grayscale:
img = frame.to_ndarray(format="bgr24")
img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
return img
webrtc_streamer(key="example", video_processor_factory=VideoFilter)
The transform
method is called for each incoming video frame, allowing for real-time processing. The processed frames are then sent back to the client for display. This setup enables the development of sophisticated video processing applications that can run directly in the browser, leveraging the power of Streamlit and WebRTC.
In conclusion, Streamlit-webrtc significantly simplifies the integration of real-time video processing into Streamlit applications. By abstracting the complexities of WebRTC and providing a straightforward API for media stream handling, developers can focus on implementing their video processing logic and creating interactive, data-driven applications.
Security and Privacy in Video Chat Applications
4.1 Implementing Authentication Mechanisms
In the realm of video chat applications, the implementation of robust authentication mechanisms is paramount to ensure that only authorized users can access the service. Streamlit Video Chat App developers must prioritize the integration of authentication protocols to safeguard user identities and prevent unauthorized access. Common authentication methods include OAuth, which leverages third-party services for verification, and JWT (JSON Web Tokens), which provide secure and compact means of user authentication. Additionally, multi-factor authentication (MFA) can be employed to add an extra layer of security, requiring users to provide two or more verification factors to gain access.
Implementing these mechanisms involves careful consideration of the user experience, ensuring that security measures do not impede the ease of use. For instance, developers can utilize Streamlit's native capabilities to create seamless login interfaces that interact with backend authentication services. Code snippet examples for integrating OAuth or JWT with Streamlit might look like the following:
import streamlit as st
from authlib.integrations.streamlit_client import OAuth
oauth = OAuth()
oauth.register(
name='google',
client_id='YOUR_CLIENT_ID',
client_secret='YOUR_CLIENT_SECRET',
authorize_url='https://accounts.google.com/o/oauth2/auth',
authorize_params=None,
access_token_url='https://accounts.google.com/o/oauth2/token',
access_token_params=None,
refresh_token_url=None,
redirect_uri='YOUR_REDIRECT_URI',
client_kwargs={'scope': 'openid profile email'},
)
user = oauth.google.authorize_access_token()
4.2 Addressing Vulnerabilities and Risks
Video chat applications are susceptible to a variety of security vulnerabilities and risks, including interception of media streams, data breaches, and unauthorized access. To mitigate these risks, developers must implement end-to-end encryption (E2EE) for media streams, ensuring that video and audio data remain private and secure from the point of origin to the destination. Furthermore, regular security audits and penetration testing are essential to identify and rectify potential security flaws within the application.
Streamlit Video Chat App developers should also be aware of the risks associated with third-party libraries and dependencies. Regularly updating these components to their latest versions can prevent exploitation of known vulnerabilities. Additionally, developers must be vigilant in monitoring for zoom-bombing—a form of cyberattack where unauthorized individuals disrupt video conferences—by implementing room access controls and user reporting mechanisms.
To exemplify the implementation of E2EE in a Streamlit application, developers might use the pycryptodome
library to encrypt and decrypt media streams:
from Crypto.Cipher import AES
from Crypto.Random import get_random_bytes
# Encryption
key = get_random_bytes(16) # AES key must be either 16, 24, or 32 bytes long
cipher = AES.new(key, AES.MODE_EAX)
nonce = cipher.nonce
ciphertext, tag = cipher.encrypt_and_digest(data)
# Decryption
cipher = AES.new(key, AES.MODE_EAX, nonce=nonce)
plaintext = cipher.decrypt_and_verify(ciphertext, tag)
Security and privacy of video chat applications like Streamlit Video Chat App hinge on the diligent implementation of authentication mechanisms and the proactive addressing of vulnerabilities and risks. By adhering to industry best practices and leveraging the latest security technologies, developers can provide users with a secure and private video chat experience.
Optimizing and Deploying to Production
5.1 Best Practices for Deployment
When preparing a Streamlit Video Chat App for deployment, it is crucial to adhere to a set of best practices to ensure a smooth transition from development to production. Firstly, the codebase should be thoroughly reviewed for any debugging or development-specific code that may hinder performance or security in a production environment. This includes removing any hardcoded sensitive information and replacing it with environment variables or secure app configurations.
Secondly, it is recommended to containerize the application using technologies such as Docker. Containerization encapsulates the application and its dependencies, ensuring consistency across different environments and simplifying the deployment process. The Dockerfile
should be optimized to reduce image size and build time, leveraging multi-stage builds and efficient caching of layers.
Lastly, continuous integration and continuous deployment (CI/CD) pipelines should be established to automate the testing and deployment processes. This automation ensures that every change to the codebase is tested and that the deployment to production is executed reliably and consistently. Tools such as GitHub Actions can be configured to run a series of automated tests and, upon successful completion, deploy the application to a cloud service provider like AWS, GCP, or Azure.
5.2 Scaling and Performance Considerations
To ensure that the Streamlit Video Chat App can handle a growing number of users and maintain high performance, scalability must be a core consideration. One approach is to implement a load balancing solution that distributes incoming traffic across multiple instances of the application. This not only improves response times but also provides redundancy in case of instance failure.
In terms of the application's architecture, it is advisable to separate compute-intensive tasks from the main application thread. Asynchronous processing and the use of background workers can help in managing long-running tasks without blocking the main thread, thus maintaining a responsive user interface.
Monitoring and logging are also pivotal for maintaining performance. By implementing a robust monitoring system, developers can track the application's health and performance metrics in real-time. This data is invaluable for identifying bottlenecks and issues as they arise, allowing for proactive optimization efforts.
Finally, it is essential to consider the database and storage solutions in use. They should be chosen and configured to handle concurrent connections and large volumes of data efficiently. Using caching mechanisms and database indexing can significantly reduce latency and improve the overall user experience.
By following these best practices and considerations, developers can ensure that their Streamlit Video Chat App is not only ready for production but also primed for scalability and optimal performance.