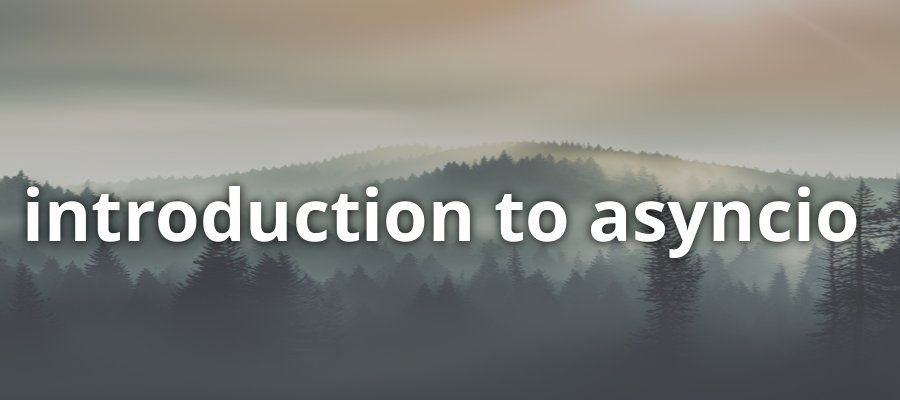
Introduction to Asyncio
• January 4, 2024
Asyncio is a Python library introduced in Python 3.5, designed to handle asynchronous I/O, event loops, and coroutines. It provides a framework for writing concurrent code using the async/await syntax.
• January 4, 2024
Asyncio is a Python library introduced in Python 3.5, designed to handle asynchronous I/O, event loops, and coroutines. It provides a framework for writing concurrent code using the async/await syntax.
Asyncio is a Python library introduced in Python 3.5, designed to handle asynchronous I/O, event loops, and coroutines. It provides a framework for writing concurrent code using the async/await syntax. Asyncio is particularly suited for IO-bound and high-level structured network code. It operates on a single-threaded event loop, where coroutines can be scheduled to run, allowing for non-blocking code execution without the need for multi-threading.
The async/await paradigm is a syntactic feature in Python that enables asynchronous programming. An async
function is defined with the async def
syntax and returns a coroutine object. Within these async
functions, await
is used to pause the execution of the coroutine, yielding control back to the event loop, until the awaited task is complete. This model allows for a more readable and maintainable structure compared to callback-based code.
async def fetch_data():
data = await some_io_task()
return data
The event loop is the core of the asyncio library. It is responsible for managing and distributing the execution of different tasks. It runs in a loop, waiting for and dispatching events to the appropriate coroutine. The event loop uses mechanisms like epoll or kqueue to efficiently wait for events on multiple channels. A fundamental understanding of the event loop is crucial for effectively leveraging asyncio's capabilities.
import asyncio
async def main():
# Coroutine that performs a task
await some_task()
# Run the event loop
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
loop.close()
Asyncio, a library introduced in Python 3.5, has become a cornerstone for writing concurrent code using the async/await syntax. This section delves into the core concepts necessary for implementing asyncio effectively, ensuring that developers can leverage its capabilities to write efficient and scalable asynchronous programs.
Native coroutines in Python are defined using the async def
syntax. These coroutines are not executed immediately; instead, they return a coroutine object which can be awaited, thus allowing the event loop to manage its execution.
async def my_coroutine():
await some_async_operation()
Tasks are a way to schedule the execution of a coroutine. When a coroutine is wrapped into a Task with functions like asyncio.create_task()
, it is then managed by the event loop, which handles its execution in the background.
task = asyncio.create_task(my_coroutine())
It is crucial to understand that while coroutines are the fundamental building blocks, tasks are the objects that are actually executed by the event loop. Tasks can be awaited, thus allowing one to retrieve the result of the coroutine's execution or handle exceptions that it might raise.
Effective use of asyncio requires adherence to certain design patterns and best practices. One such pattern is the proper handling of cancellation. Tasks in asyncio can be cancelled, which raises a CancelledError
in the task's coroutine. It is essential for coroutines to be designed to handle this exception, ensuring resources are released properly.
Another best practice is to avoid blocking operations within coroutines. This includes I/O operations or CPU-bound tasks that can halt the progress of the event loop. Instead, such operations should be run in a thread or process pool using functions like asyncio.to_thread()
or asyncio.run_in_executor()
.
result = await asyncio.to_thread(blocking_io_operation)
Utilizing context managers for resource management within coroutines is also recommended. This ensures that resources are properly managed even when exceptions or cancellations occur.
The event loop is the core of asyncio's execution model. It is responsible for running asynchronous tasks, handling I/O events, and managing subprocesses. Developers must understand how to manage the event loop to ensure their applications run smoothly.
Creating an event loop is typically done using asyncio.new_event_loop()
, and setting it as the current loop with asyncio.set_event_loop()
. However, in most scenarios, the default event loop provided by the asyncio runtime is sufficient.
loop = asyncio.new_event_loop()
asyncio.set_event_loop(loop)
Running the event loop is accomplished with loop.run_until_complete()
, which will run until the given coroutine is complete, or loop.run_forever()
, which will run until loop.stop()
is called.
It is important to note that the event loop should not be accessed from different threads unless it is thread-safe, which is typically not the case. Special care must be taken when dealing with asynchronous code that interacts with multi-threaded environments.
In conclusion, understanding and implementing the core concepts of asyncio, such as native coroutines, tasks, design patterns, and event loop management, are fundamental for developers to effectively use asyncio in their Python applications. These concepts form the foundation upon which robust and efficient asynchronous programs are built.
Asynchronous programming in Python is facilitated by the asyncio
library, which provides the necessary infrastructure to write concurrent code using coroutines, event loops, and futures. This section delves into the practical aspects of building asynchronous programs using asyncio
.
asyncio
is a Python library introduced in version 3.4 that allows for writing concurrent code using an asynchronous model. It is particularly well-suited for I/O-bound and high-level structured network code. The library enables the execution of code in an event-driven manner, where the flow of the program is determined by events such as the completion of I/O operations.
The async/await syntax introduced in Python 3.5 is a declarative way of defining asynchronous functions. An async
function is a coroutine that can be paused and resumed at await points, allowing other coroutines to run.
async def fetch_data():
data = await some_io_task()
return data
In this example, fetch_data
is a coroutine that awaits the completion of an I/O task before proceeding. The await
keyword suspends the execution of fetch_data
until some_io_task
is finished, allowing the event loop to run other tasks in the meantime.
The event loop is the core of the asyncio
library. It is responsible for managing and distributing the execution of different tasks. It keeps track of all the running coroutines and executes them when they are ready, ensuring that I/O operations do not block the flow of the program.
import asyncio
async def main():
# Schedule coroutine execution
await fetch_data()
# Get the default event loop
loop = asyncio.get_event_loop()
# Run the main coroutine
loop.run_until_complete(main())
In this snippet, main
is a coroutine that the event loop executes. The run_until_complete
method of the event loop runs main
until it is finished.
Error handling in asynchronous programs follows similar principles to synchronous code but requires attention to the unique behavior of coroutines and tasks.
When a coroutine raises an exception, it propagates to the point where the coroutine was awaited. If unhandled, it can cause the task to fail. To handle exceptions in coroutines, use try-except blocks.
async def fetch_data():
try:
data = await some_io_task()
return data
except IOError as e:
# Handle I/O errors
log_error(e)
In this coroutine, fetch_data
handles IOError
exceptions that may occur during the I/O task.
It is crucial to follow best practices when handling errors in asynchronous programs. This includes proper exception handling, using timeouts to avoid hanging coroutines, and cleaning up resources in a finally
block.
async def fetch_data_with_timeout():
try:
# Wait for at most 5 seconds
return await asyncio.wait_for(some_io_task(), timeout=5)
except asyncio.TimeoutError:
# Handle the case where the I/O task took too long
handle_timeout()
finally:
# Perform any necessary cleanup
cleanup_resources()
This coroutine, fetch_data_with_timeout
, demonstrates the use of a timeout to prevent the coroutine from waiting indefinitely for an I/O task.
Proper management of the event loop includes handling exceptions that occur during the execution of tasks and ensuring that the loop is closed cleanly when the program is finished.
try:
loop.run_until_complete(main())
except Exception as e:
# Handle exceptions that occurred during event loop execution
log_error(e)
finally:
loop.close()
This code block shows how to run the event loop with exception handling and ensures that the loop is closed in a finally
block.
By understanding and implementing these practical examples, developers can effectively use asyncio
to build robust and efficient asynchronous programs in Python.
Asyncio is inherently single-threaded, utilizing an event loop to manage asynchronous tasks. However, certain scenarios necessitate the integration of asyncio with multithreading or multiprocessing to leverage parallelism, especially for CPU-bound tasks or for running blocking I/O code concurrently.
While asyncio's event loop runs in a single thread, it can be beneficial to use threads to perform blocking I/O operations that would otherwise stall the event loop. The concurrent.futures.ThreadPoolExecutor
allows for the execution of I/O-bound tasks in separate threads, integrating with asyncio through the loop.run_in_executor()
method.
import asyncio
from concurrent.futures import ThreadPoolExecutor
async def main():
loop = asyncio.get_running_loop()
with ThreadPoolExecutor() as pool:
result = await loop.run_in_executor(pool, blocking_io_function)
print('Blocking I/O function result:', result)
asyncio.run(main())
For CPU-bound operations, multiprocessing is a more suitable approach. Asyncio can work with the concurrent.futures.ProcessPoolExecutor
to offload intensive computations to separate processes, thus avoiding blocking the event loop.
import asyncio
from concurrent.futures import ProcessPoolExecutor
async def main():
loop = asyncio.get_running_loop()
with ProcessPoolExecutor() as pool:
result = await loop.run_in_executor(pool, cpu_bound_function)
print('CPU-bound function result:', result)
asyncio.run(main())
Asyncio can be integrated with other Python libraries to create highly efficient and scalable applications. However, care must be taken to ensure that these libraries are compatible with asyncio's asynchronous execution model or can be adapted to work within an asynchronous context.
Many existing Python libraries are synchronous and can block the event loop. To maintain the non-blocking nature of asyncio, it is often necessary to run synchronous code in a separate thread or process, as previously discussed.
Some libraries offer asynchronous support or can be wrapped in an asynchronous interface. For example, databases that support asynchronous communication can be queried using async/await syntax, and HTTP requests can be made asynchronously using libraries like aiohttp
.
import aiohttp
import asyncio
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
async with aiohttp.ClientSession() as session:
html = await fetch(session, 'http://python.org')
print(html)
asyncio.run(main())
In conclusion, asyncio's integration with multithreading, multiprocessing, and other Python libraries expands its utility beyond simple asynchronous I/O tasks, making it a powerful tool for a wide range of concurrent programming scenarios.
In the realm of concurrent programming within Python, asyncio
stands as a pivotal framework, enabling developers to write code that is not only efficient but also non-blocking. This efficiency is achieved through the use of asynchronous programming patterns, which allow for the execution of multiple tasks seemingly in parallel, despite operating on a single thread.
The utilization of asyncio
can significantly enhance the performance of an application, particularly in I/O-bound and high-level structured network code. By employing the async/await
syntax, developers can maintain readable and maintainable code, while also ensuring that the underlying event loop efficiently manages the execution of coroutines.
To fully leverage the power of asyncio
, it is imperative to understand the core concepts such as event loops, coroutines, tasks, and futures. Mastery of these concepts allows for the crafting of high-performance applications that can handle a multitude of simultaneous I/O-bound tasks without the overhead of multi-threading.
In conclusion, asyncio
is a robust library that, when correctly implemented, can significantly reduce latency and increase throughput in Python applications. Its design encourages developers to think concurrently, leading to the development of high-performance applications that are well-suited to the demands of modern software solutions.